Java_beginner(Repl.it)
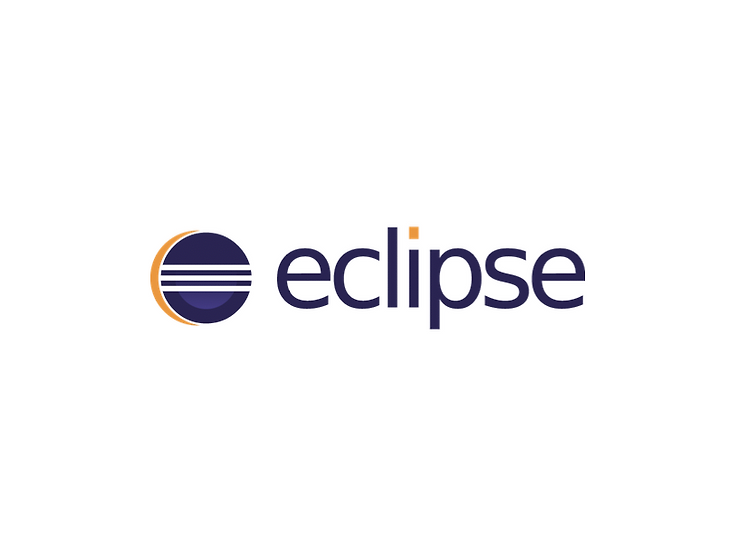
059 - Scope and Global Variables 2
Within the main class, create three class variables: A boolean variable called bool with a value of false A String variable called str with a value of "sup" An integer variable called i with a value of 10 Solution 1 2 3 4 5 6 7 8 9 10 class Main { boolean bool=false; String str="sup"; int i =10; public static void main(String[] args) { System.out.println("nothing to do here..."); } }
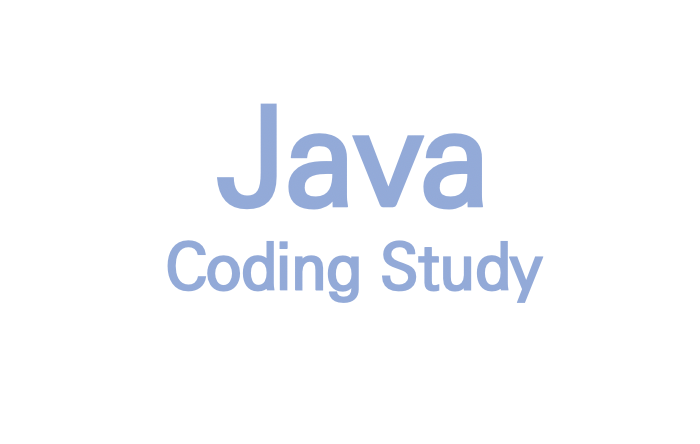
058 - Scope and Global Variables 1
Fix the following code so it produces the following output: one word Note: You should achieve this WITHOUT changing any print statements. Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 import java.util.*; class Main { public static void main(String[] args) { String output =""; String text = "hello"; if (text.indexOf(" ") == -1) //if a space doesn't exist { output = "one word"; } else {..
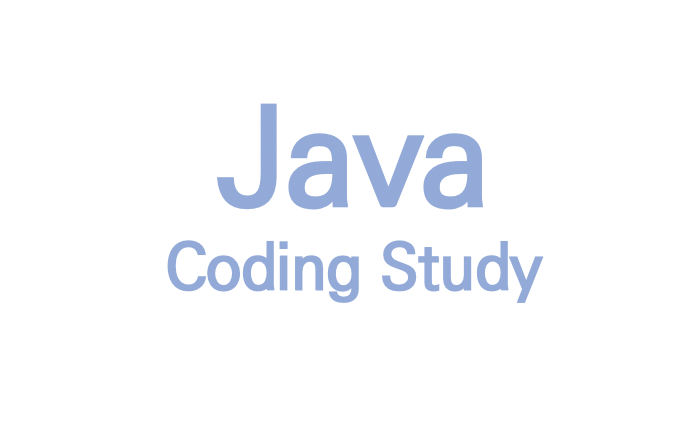
057 - Accumulator Method String Challenge 1 (optional)
Write a method header on line two with the following specs: Returns: a String Name: surroundStr Parameters: a String called s a String called search_term Then complete the method by programming the following behavior Return a new String built from s that has every instance of the search term surrounded by parentheses See below examples. Examples: surroundStr("abcabcabc","abc") ==> "(abc)(abc)(ab..
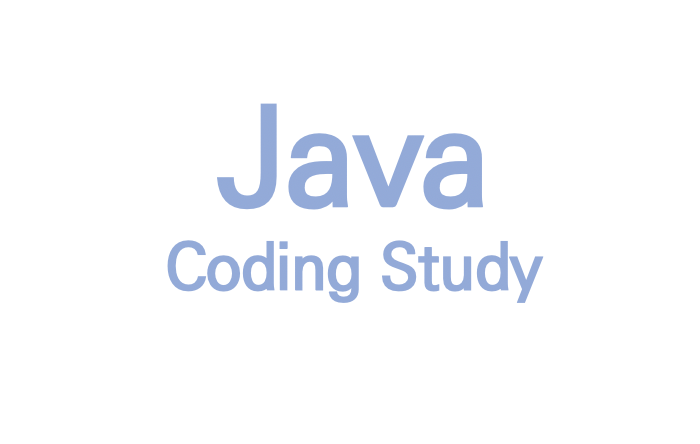
056 - Accumulator Method String Practice 4
Write a method header on line two with the following specs: Returns: a String Name: surround Parameters: a String called s a char called search_term Then complete the method by programming the following behavior Return a new String built from s that has every instance of the search term surrounded by parentheses See below examples. Examples: surround("abcabcabc",'c') ==> "ab(c)ab(c)ab(c)" surrou..
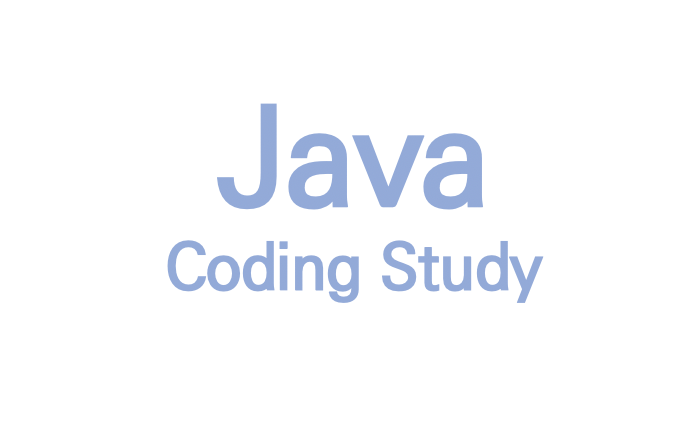
055 - Accumulator Method String Practice 3
Write a method header on line two with the following specs: Returns: a String Name: censorLetter Parameters: a String called s a char called ch Then complete the method by programming the following behavior Replace all instances of ch with a "*" within the String s. See below examples. Examples: censorLetter("computer science",'e') ==> "comput*r sci*nc*" censorLetter("trick or treat",'t') ==> "*..
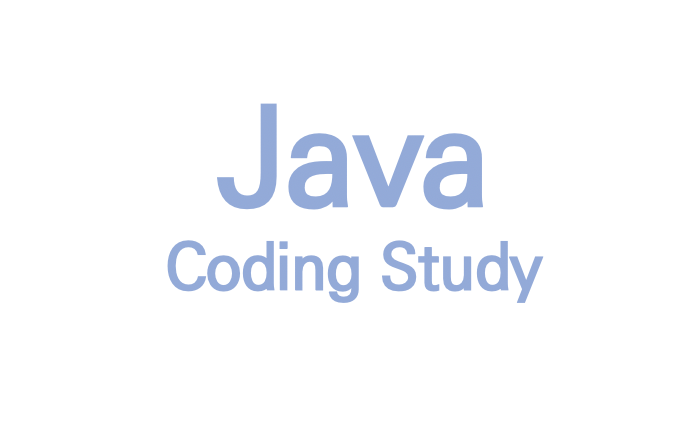
054 - Accumulator Method String Practice 2
Write a method header on line two with the following specs: Returns: a String Name: thirdLetter Parameters: a String called s Then complete the method by programming the following behavior Returns every 3rd letter of the String s, starting the first letter. See below examples. Examples: thirdLetter("hello there") ==> "hltr" thirdLetter("technology") ==> "thly" Solution 1 2 3 4 5 6 7 8 9 10 11 12..
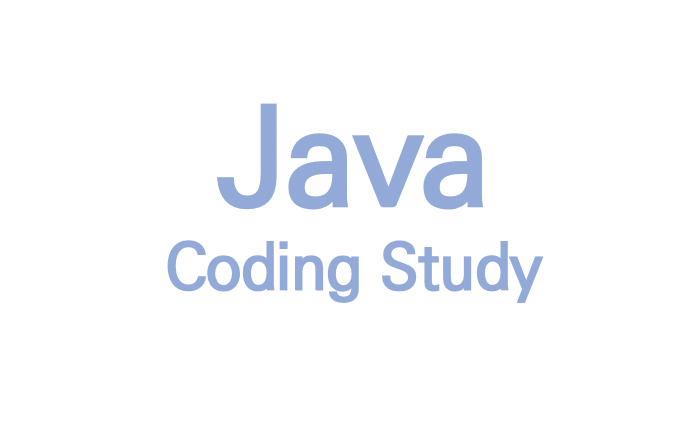
053 - Accumulator Method String Practice 1
Write a method header on line two with the following specs: Returns: a String Name: spaceOut Parameters: a String called s Then complete the method by programming the following behavior Insert spaces after every character in the String s, then return the new string. See below examples (note the space at the end as well). Examples: spaceOut("hello") ==> "h e l l o " spaceOut("technology") ==> "t ..
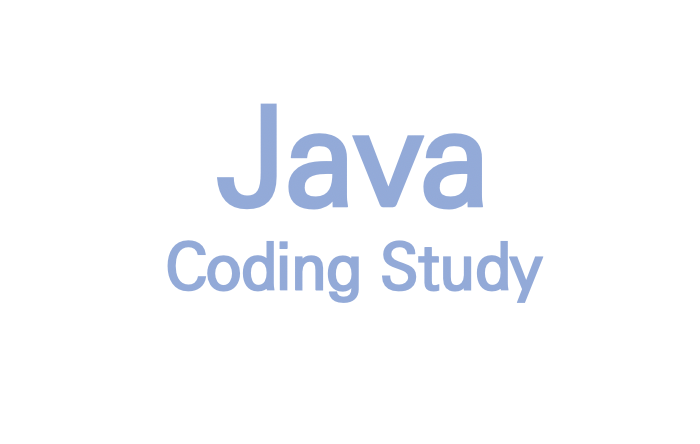
052 - Accumulator Method Challenge 2 (optional)
Write a method header on line two with the following specs: Returns: a String Name: alphabetical Parameters: a String called str Purpose: Return a string that is composed of each letter as long as the letter is later on in the alphabet than its previous one. You can assume actual parameters are lowercase. See below examples. Additional Info: You can use to compare characters (not Strings..
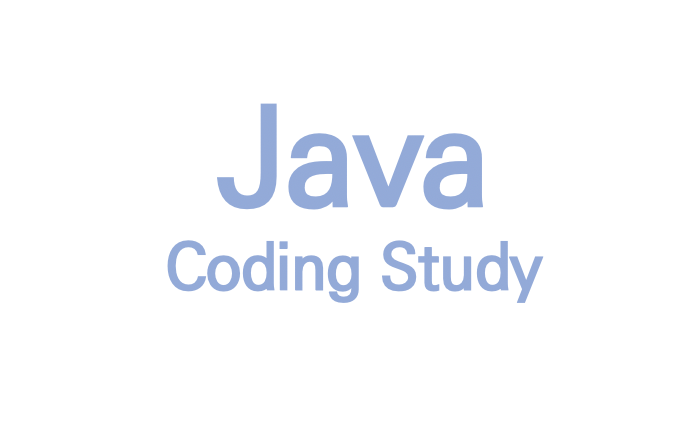
051 - Accumulator Method Challenge 1 (optional)
Write a method header on line two with the following specs: Returns: an integer Name: countString Parameters: a String called str a String called toFind Purpose: Count the number of occurrences of toFind within str. See below examples. Examples: countString("crazy crayfish crushing craniums", "cra") ==> 3 countString("sometimes solutions dont come on time", "me") ==> 4 Solution 1 2 3 4 5 6 7 8 9..
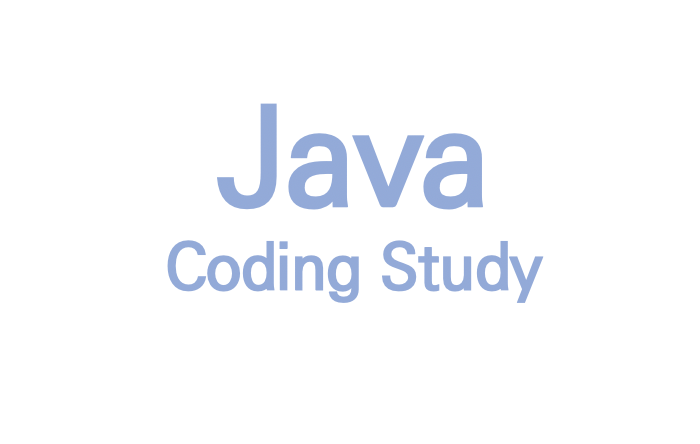
050 - Accumulator Method Practice 6
Write a method header on line two with the following specs: Returns: a String Name: keepVowels Parameters: a String called s Purpose: create a string that is composed of all the vowels in the string, in the same order that they appear. see below examples for clarity. Examples: keepVowels("hello") ==> "eo" keepVowels("how do i internets") ==> "ooiiee" Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 ..
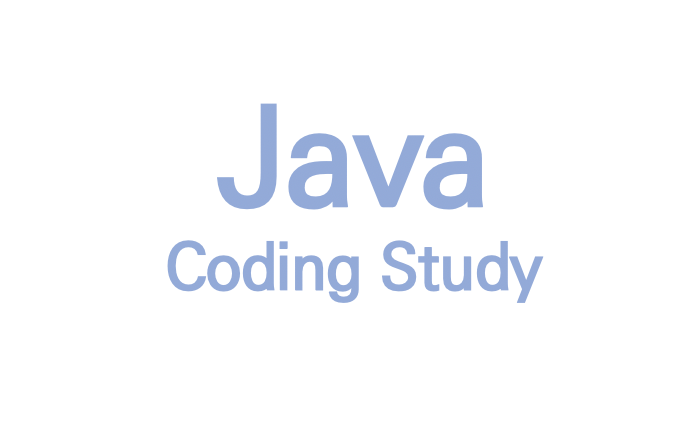
049 - Accumulator Method Practice 5
Write a method header on line two with the following specs: Returns: an integer Name: countVowels Parameters: a String called s Purpose: count the number of vowels in the string s. Assume s is all lowercase. Examples: countVowels("obama") ==> 3 countVowels("happy friday! i love weekends") ==> 9 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 class Main { public static int..
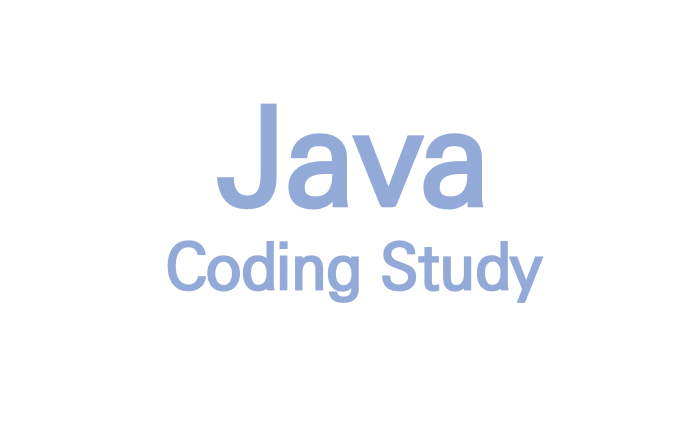
048 - Accumulator Method Practice 4
Write a method header on line two with the following specs: Returns: an integer Name: countA Parameters: a String called s Purpose: count the number of occurrences of 'a' or 'A' within s Examples: countA("aaa") ==> 3 countA("aaBBdf8k3AAadnklA") ==> 6 Hint: How do you write a for loop to loop through every letter of a string? You've done this multiple times already :) 1 2 3 4 5 6 7 8 9 10 11 12 1..
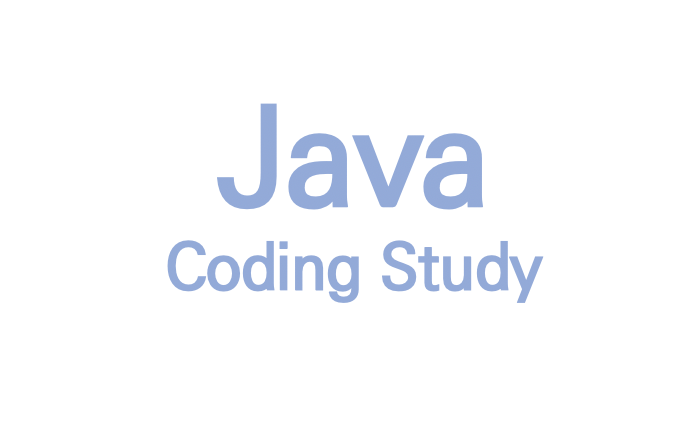
047- Accumulator Method Practice 3
Write a method header on line two with the following specs: Returns: an integer Name: sumFivesRange Parameters: an integer called "a" that represents the beginning of the range an integer called "b" that represents the end of the range Purpose: calculate the sum of the multiples of 5 within the range a to b inclusive (including b) Examples: sumFivesRange(5,15) ==> 30 sumFivesRange(11,28) ==> 60 ..
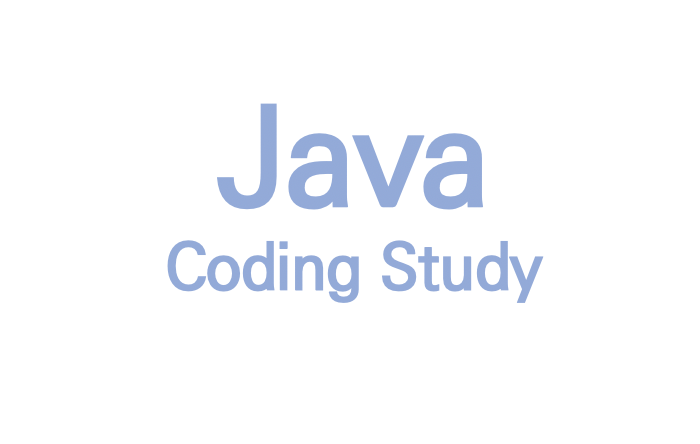
046 - Accumulator Method Practice 2
Write a method header on line two with the following specs: Returns: an integer Name: sumEvenToX Parameters: an integer called "x" Purpose: calculate the sum of the EVEN integers from 1 to x (including x) Examples: sumEvenToX(5) ==> 6 sumEvenToX(8) ==> 20 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 import java.io.*; import java.util.*; import java.util.stream.*; class Main { public ..
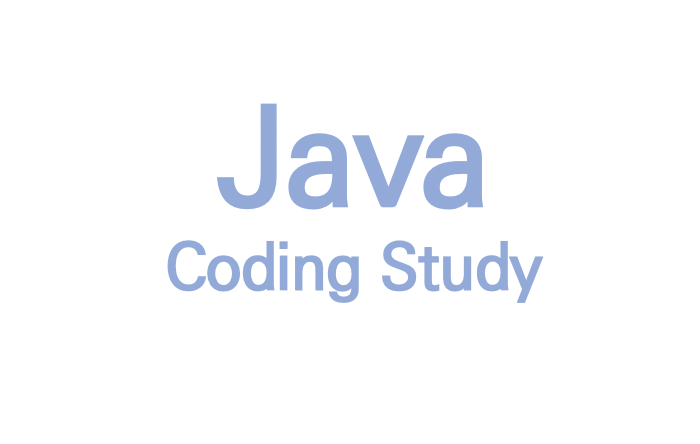
045 - Accumulator Method Practice 1
Write a method header on line two with the following specs: Returns: an integer Name: sumToX Parameters: an integer called "x" Purpose: calculate the sum of the integers from 1 to x (including x) Examples: sumToX(5) ==> 15 sumToX(7) ==> 28 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 import java.util.*; class Main { public static int sumToX(int x) { int sum=0; for(int i=1;i
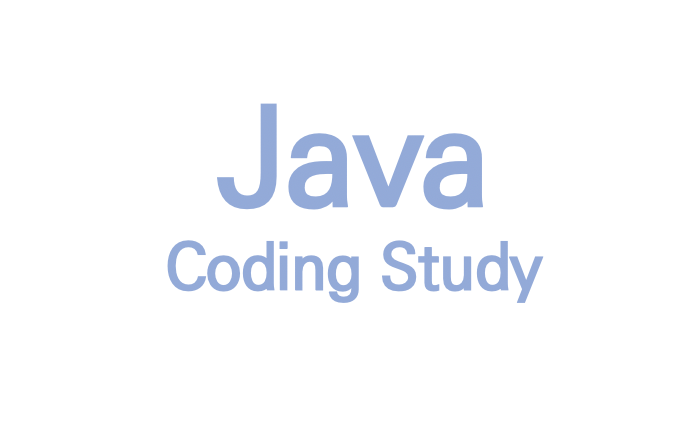
044 - Method Header Challenge 1 (Optional)
Write a method header on line two with the following specs: Returns: a String Name: mixString Parameters: a String called s1 a String called s2 Then, starting on line 4, write code that will return the strings combined, one letter at a time, starting with s1. See example below for an example. You can assume that s1 and s2 are equal lengths: s1 ==> "12345" s2 ==> "abcde" mixString(s1,s2) ==> "1a2..
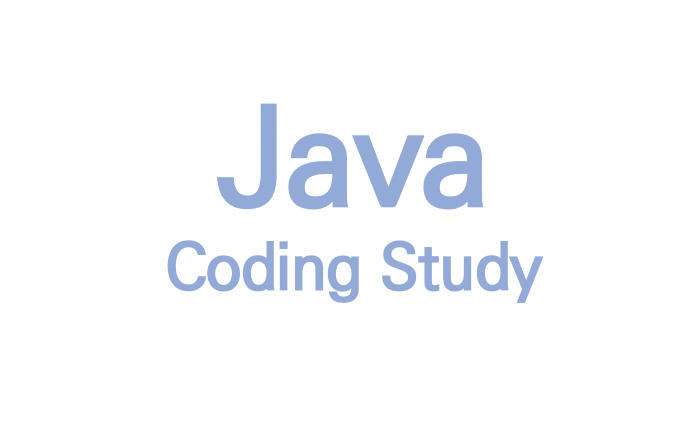
043 - Method Header Practice 8
Write a method header on line two with the following specs: Returns: a String Name: makeThreeSubstr Parameters: a String called "word" an integer called "startIndex" an integer called "endIndex" Then, starting on line 4, write code that will return 3x the substring (no spaces) from "startIndex" to "endIndex" Examples: makeThreeSubstr("hello",0,2) ==> "hehehe" makeThreeSubstr("shenanigans",3,7) =..
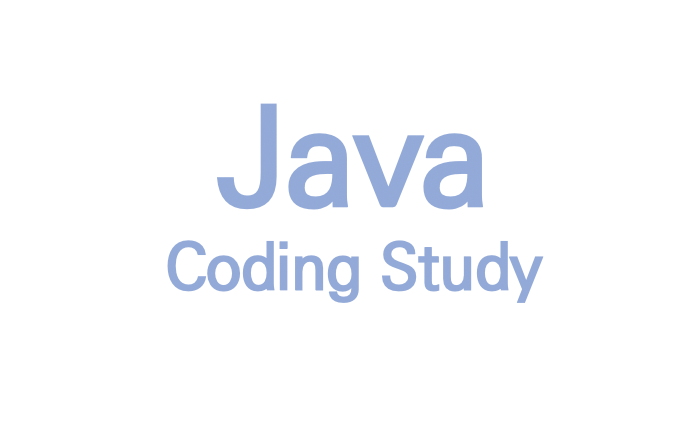
042 - Method Header Practice 7
Write a method header on line two with the following specs: Returns: a char Name: getChar Parameters: a String called "word" an integer called "index" Then, starting on line 4, write code that will return the character in "word" at the index "index" Examples: getChar("hello",1) ==> 'e' 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; class Main { public static char getChar(String word, int index) ..
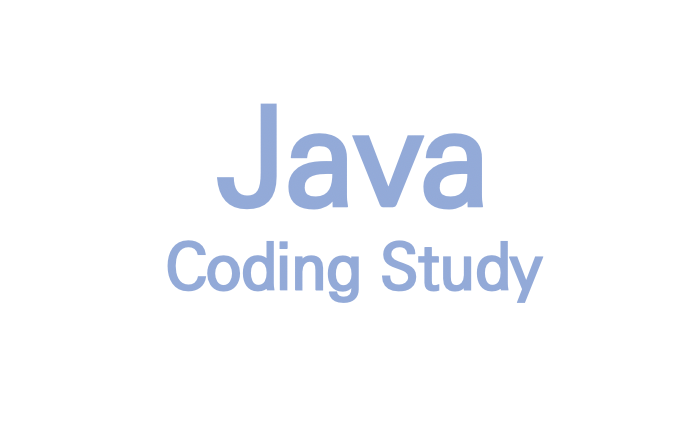
041 - Method Header Practice 6
Write a method header on line two with the following specs: Returns: a boolean Name: bothEven Parameters: an integer called "num1" an integer called "num2" Then, starting on line 4, write code that will return true if both num1 and num2 are even. Return false otherwise. Examples: bothEven(4,6) ==> true bothEven(3,4) ==> false bothEven(-1,1) ==> false 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.uti..
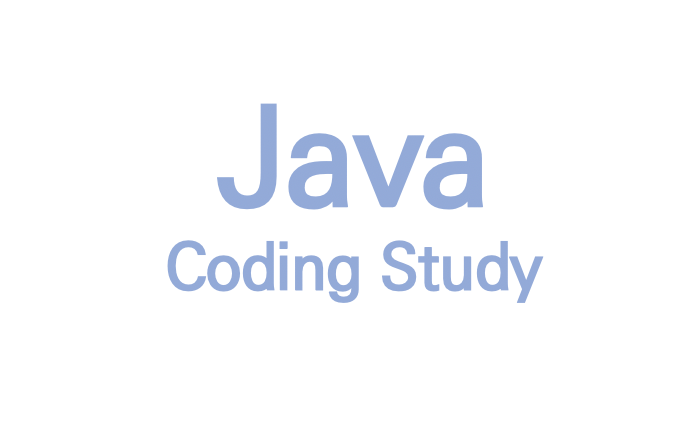
040 - Method Header Practice 5
Write a method header on line two with the following specs: Returns: a double Name: negate Parameters: a double called "num" Then, starting on line 4, write the code that will return the opposite value of num (if it's positive, make it negative, otherwise make it positive) Examples: 4 ==> -4 -10 ==> 10 1 ==> -1 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.util.*; class Main { public static..