java basic
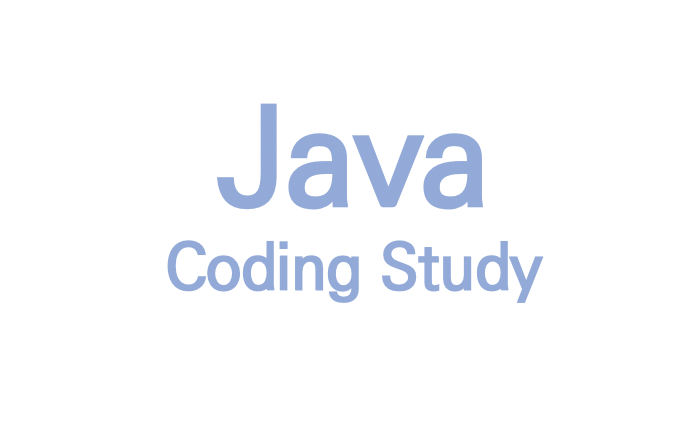
057 - Accumulator Method String Challenge 1 (optional)
Write a method header on line two with the following specs: Returns: a String Name: surroundStr Parameters: a String called s a String called search_term Then complete the method by programming the following behavior Return a new String built from s that has every instance of the search term surrounded by parentheses See below examples. Examples: surroundStr("abcabcabc","abc") ==> "(abc)(abc)(ab..
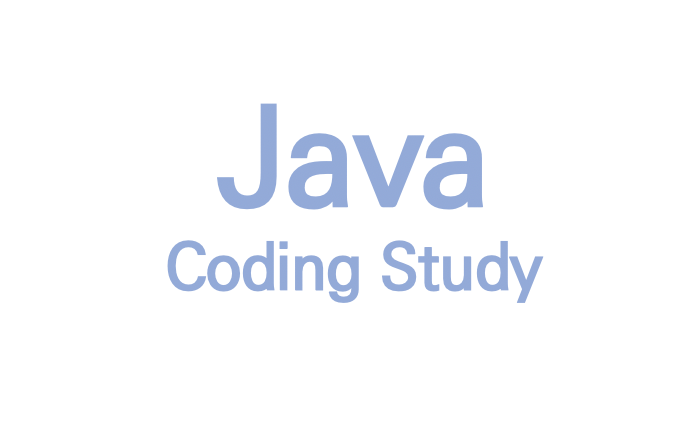
055 - Accumulator Method String Practice 3
Write a method header on line two with the following specs: Returns: a String Name: censorLetter Parameters: a String called s a char called ch Then complete the method by programming the following behavior Replace all instances of ch with a "*" within the String s. See below examples. Examples: censorLetter("computer science",'e') ==> "comput*r sci*nc*" censorLetter("trick or treat",'t') ==> "*..
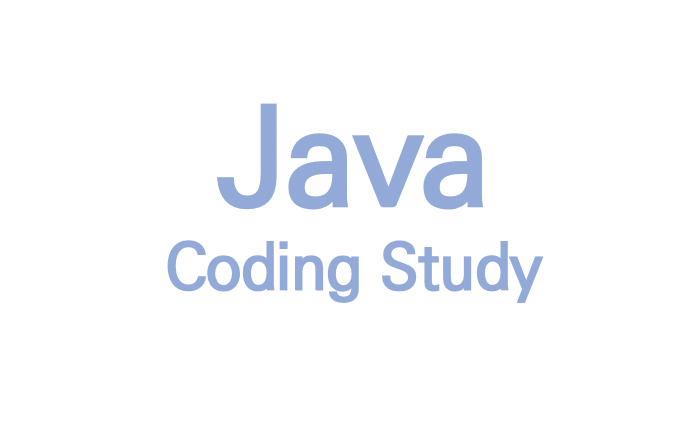
053 - Accumulator Method String Practice 1
Write a method header on line two with the following specs: Returns: a String Name: spaceOut Parameters: a String called s Then complete the method by programming the following behavior Insert spaces after every character in the String s, then return the new string. See below examples (note the space at the end as well). Examples: spaceOut("hello") ==> "h e l l o " spaceOut("technology") ==> "t ..
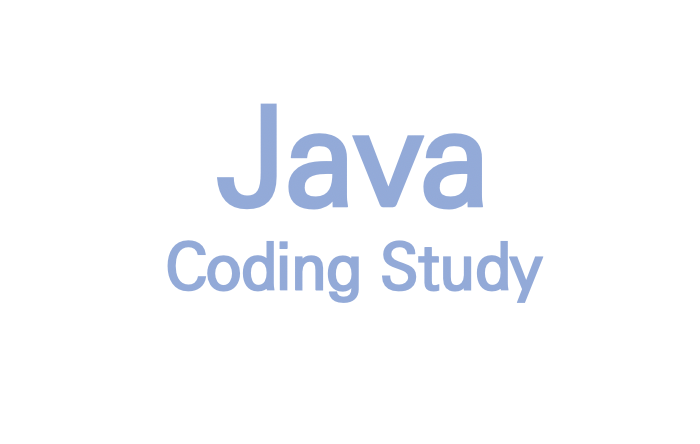
052 - Accumulator Method Challenge 2 (optional)
Write a method header on line two with the following specs: Returns: a String Name: alphabetical Parameters: a String called str Purpose: Return a string that is composed of each letter as long as the letter is later on in the alphabet than its previous one. You can assume actual parameters are lowercase. See below examples. Additional Info: You can use to compare characters (not Strings..
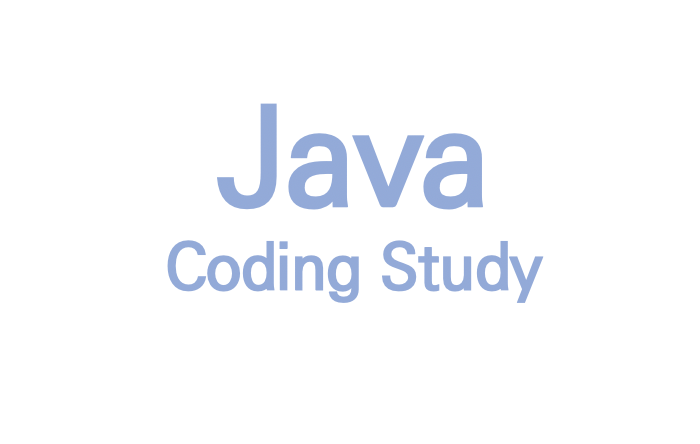
050 - Accumulator Method Practice 6
Write a method header on line two with the following specs: Returns: a String Name: keepVowels Parameters: a String called s Purpose: create a string that is composed of all the vowels in the string, in the same order that they appear. see below examples for clarity. Examples: keepVowels("hello") ==> "eo" keepVowels("how do i internets") ==> "ooiiee" Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 ..
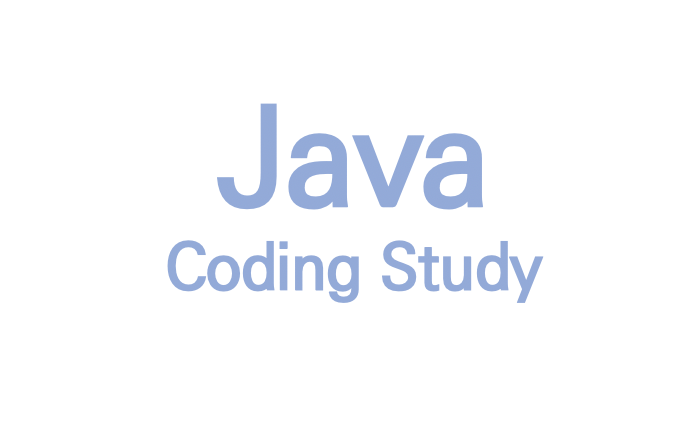
049 - Accumulator Method Practice 5
Write a method header on line two with the following specs: Returns: an integer Name: countVowels Parameters: a String called s Purpose: count the number of vowels in the string s. Assume s is all lowercase. Examples: countVowels("obama") ==> 3 countVowels("happy friday! i love weekends") ==> 9 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 class Main { public static int..
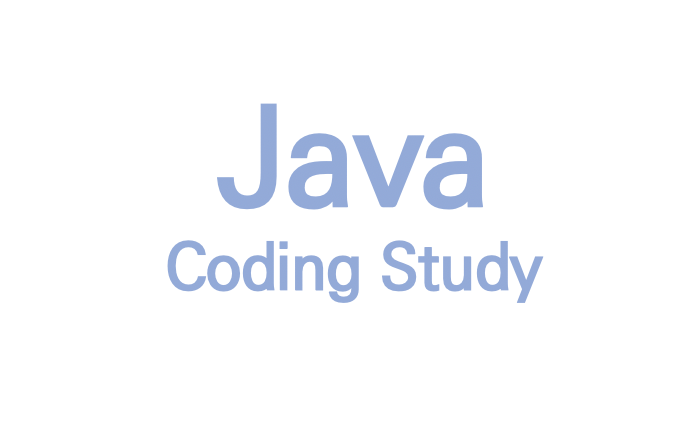
047- Accumulator Method Practice 3
Write a method header on line two with the following specs: Returns: an integer Name: sumFivesRange Parameters: an integer called "a" that represents the beginning of the range an integer called "b" that represents the end of the range Purpose: calculate the sum of the multiples of 5 within the range a to b inclusive (including b) Examples: sumFivesRange(5,15) ==> 30 sumFivesRange(11,28) ==> 60 ..
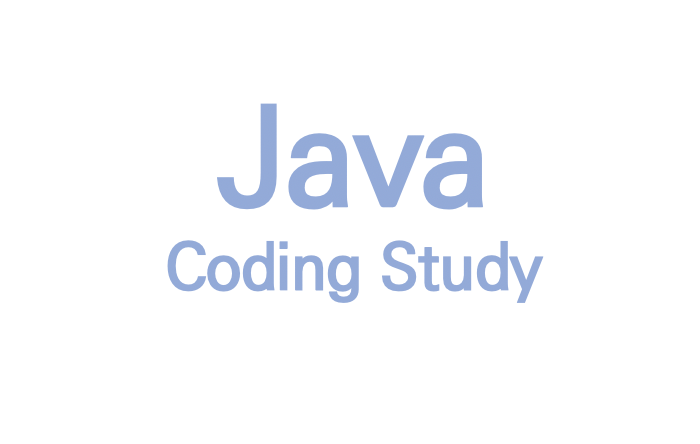
046 - Accumulator Method Practice 2
Write a method header on line two with the following specs: Returns: an integer Name: sumEvenToX Parameters: an integer called "x" Purpose: calculate the sum of the EVEN integers from 1 to x (including x) Examples: sumEvenToX(5) ==> 6 sumEvenToX(8) ==> 20 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 import java.io.*; import java.util.*; import java.util.stream.*; class Main { public ..
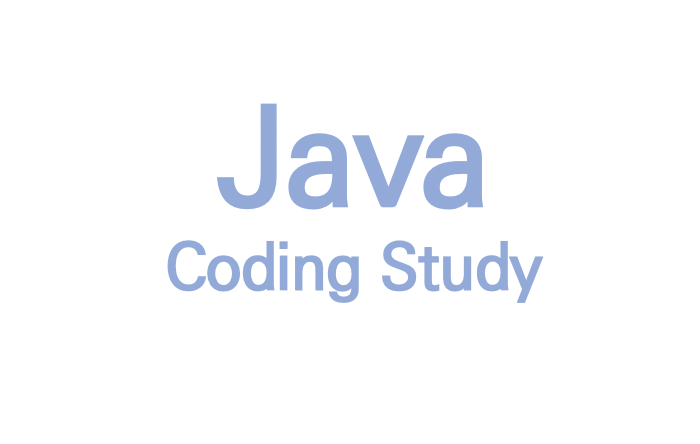
045 - Accumulator Method Practice 1
Write a method header on line two with the following specs: Returns: an integer Name: sumToX Parameters: an integer called "x" Purpose: calculate the sum of the integers from 1 to x (including x) Examples: sumToX(5) ==> 15 sumToX(7) ==> 28 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 import java.util.*; class Main { public static int sumToX(int x) { int sum=0; for(int i=1;i
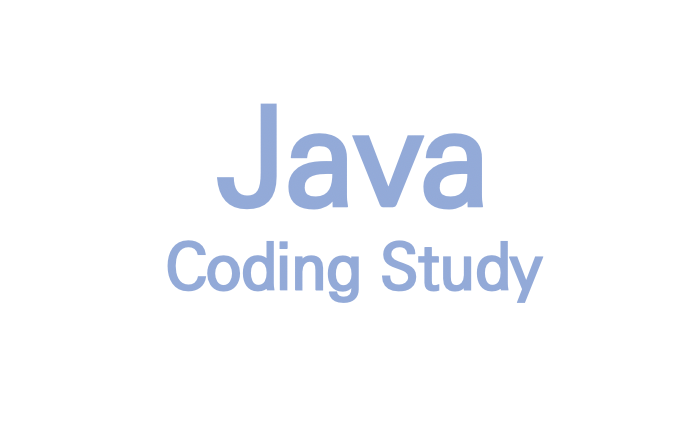
041 - Method Header Practice 6
Write a method header on line two with the following specs: Returns: a boolean Name: bothEven Parameters: an integer called "num1" an integer called "num2" Then, starting on line 4, write code that will return true if both num1 and num2 are even. Return false otherwise. Examples: bothEven(4,6) ==> true bothEven(3,4) ==> false bothEven(-1,1) ==> false 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.uti..
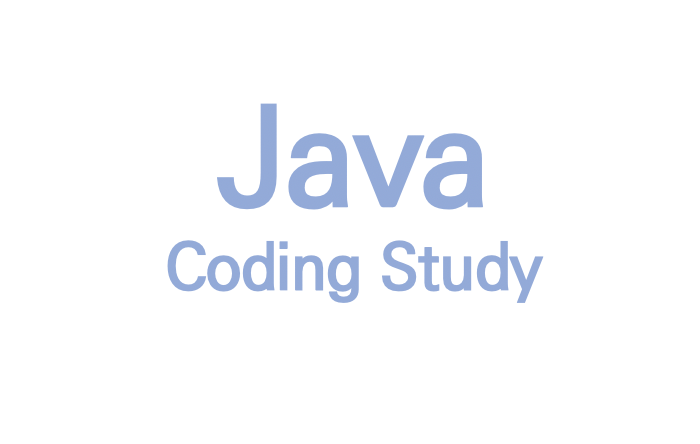
039 - Method Header Practice 4
Write a method header on line two with the following specs: Returns: an integer Name: addTwo Parameters: An integer called "x" An integer called "y" You should not be writing code on any line other than #2 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; class Main { public static int addTwo(int x,int y){ return x+y; } //test case below (dont change): public static void main(String[] args){ System..
![[백준/5586번] JOI와 IOI(JOI 2008)[Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FnQpYC%2FbtqApHkommu%2FY7u1S6E1w9SeMwcMF5ycb1%2Fimg.png)
[백준/5586번] JOI와 IOI(JOI 2008)[Java]
문제 입력으로 주어지는 문자열에서 연속으로 3개의 문자가 JOI 또는 IOI인 곳이 각각 몇 개 있는지 구하는 프로그램을 작성하시오. 문자열을 알파벳 대문자로만 이루어져 있다. 예를 들어, 아래와 같이 "JOIOIOI"에는 JOI가 1개, IOI가 2개 있다. 입력 첫째 줄에 알파벳 10000자 이내의 문자열이 주어진다. 출력 첫째 줄에 문자열에 포함되어 있는 JOI의 개수, 둘째 줄에 IOI의 개수를 출력한다. 풀이 문자열의 앞에서 부터 하나씩 , J가 나올시 O,I가 다음 열에 나오는지 확인하고 맞으면 cnt++해준다. 똑같은 방식으로 IOI도 반복해준뒤 출력해주면 완료. ㅇㅅㅇ 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26..
![[백준/5613번] 계산기 프로그램(JOI 2006) [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FdGI2fj%2FbtqAm3BCa9r%2FtzwFzWOkLwjvh1mgtFB3w0%2Fimg.png)
[백준/5613번] 계산기 프로그램(JOI 2006) [Java]
문제 덧셈, 뺄셈, 곱셈, 나눗셈을 할 수 있는 계산기 프로그램을 만드시오. 입력 입력의 각 줄에는 숫자와 +, -, *, /, =중 하나가 교대로 주어진다. 첫 번째 줄은 수이다. 연산자의 우선 순위는 생각하지 않으며, 입력 순서대로 계산을 하고, =가 주어지면, 그때까지의 결과를 출력한다. 주어지는 수는 108 이하의 양의 정수이다. 계산 중 결과는 0 또는 음수가 될 수 있지만, -108 ~ 108 범위를 넘지는 않는다. 또, 나눗셈에서 소수점은 버린다. 따라서, 100/3*3 = 99이다. 출력 첫째 줄에 계산 결과를 출력한다. 풀이 입력을 어떻게 받을까 고민하다가 우선 첫 숫자는 무조건 숫자이므로 받고 이후 문자, 정수값으로 번갈아가면서 받는 것으로 구상하였다. while문을 통해서 문자열값에 ..
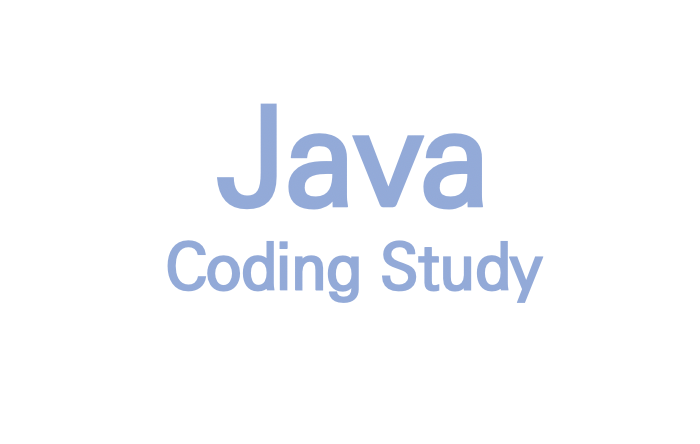
038 - Method Header Practice 3
Write a method header on line two with the following specs: Returns: a String Name: makeCapital Parameters: a String named "name" You should not be writing code on any line other than #2 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.*; class Main { public static String makeCapital(String name){ return name.toUpperCase(); } //test case below (dont change): public static void Bakjoon(..
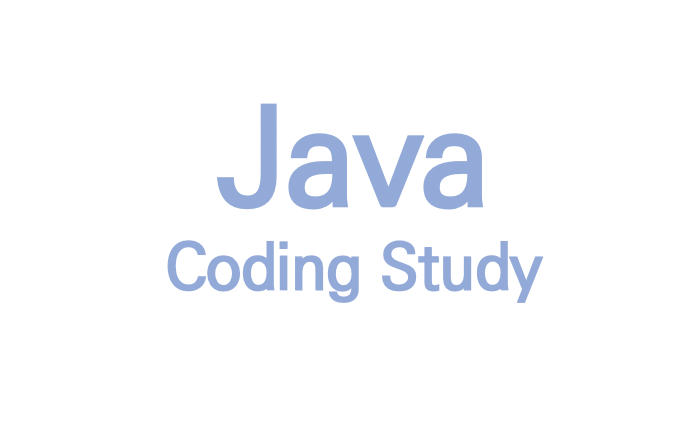
036 - Method Header Practice 1,2
036 - Method Header Practice 1 Write a method header on line two with the following specs: Returns: an integer Name: practiceOne Parameters: none You should not be writing code on any line other than #2 Solution 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; public class Main { public int practiceOne(){ { return 2; } } } 037 - Method Header Practice 2 Write a method header on line two with the f..
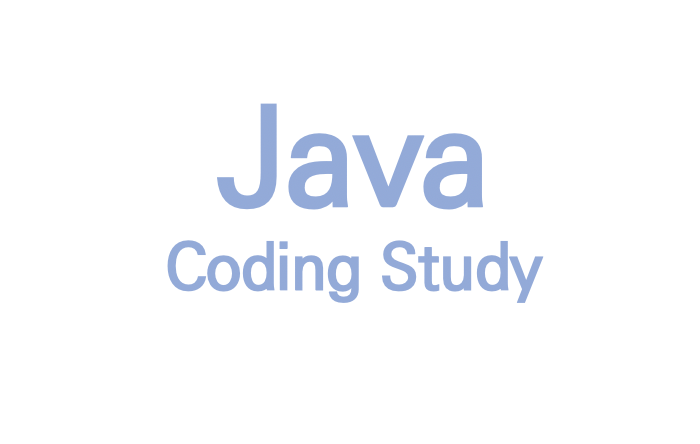
035 - Sum Odds in Range
You are given two inputs: int num1; int num2; You can assume that the following: num2 > num1 ==> TRUE You are to write a program that will calculate and print out the SUM OF ODD numbers in between num1 and num2 inclusive. Your output should all be on one line, separated by spaces. Sample input/output: #1: 3 #2: 11 Output: 35 #1: 4 #2: 20 Output: 96 #1: -2 #2: 6 Output: 8 Solution 1 2 3 4 5 6 7 8..
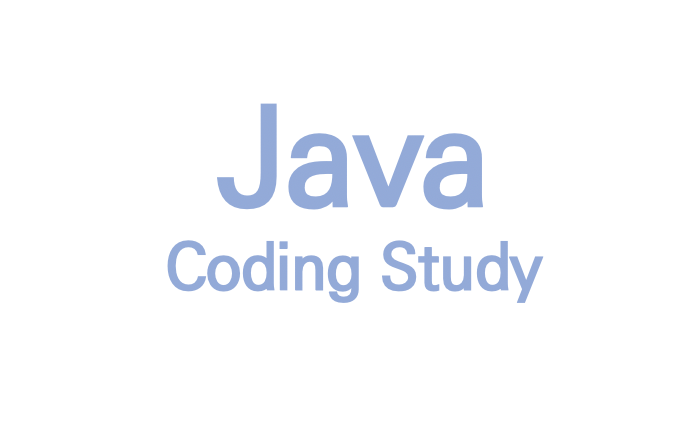
034 - Big Number Program (From Decoding)
You are given two inputs: int num1; int num2; You can assume that the following: num2 > num1 ==> TRUE You are to write a program that will print out all the ODD numbers in between num1 and num2 inclusive. Your output should all be on one line, separated by spaces. Sample input/output: #1: 3 #2: 11 3 5 7 9 11 #1: 4 #2: 20 5 7 9 11 13 15 17 19 #1: -2 #2: 6 -1 1 3 5 Solution 1 2 3 4 5 6 7 8 9 10 11..
![[백준/17009번] Winning Score ( CCC 2019 Junior Division)[Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2F3dd31%2FbtqAjnOlTpy%2FjKpANghadRKqlaTfXYMbP1%2Fimg.png)
[백준/17009번] Winning Score ( CCC 2019 Junior Division)[Java]
Problem You record all of the scoring activity at a basketball game. Points are scored by a 3-point shot, a 2-point field goal, or a 1-point free throw. You know the number of each of these types of scoring for the two teams: the Apples and the Bananas. Your job is to determine which team won, or if the game ended in a tie. Input The first three lines of input describe the scoring of the Apples,..
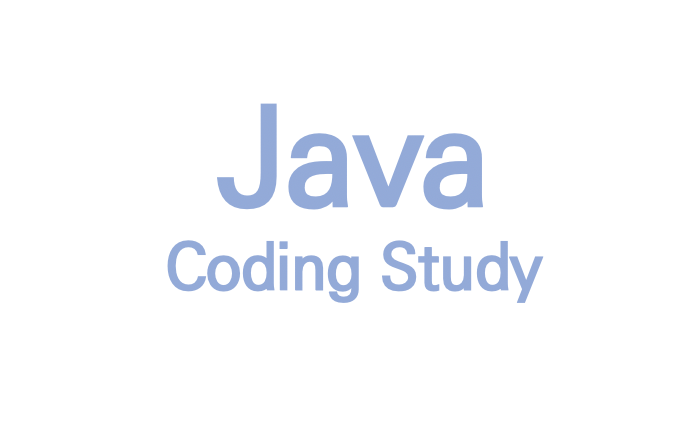
033 - For Loop Challenge 2 (optional)
Write code that will take in a String input and check to see if it is a palindrome or not. A palindrome means that the characters are the same forwards and backwards, ignoring spaces. Examples of palindromes: racecar was it a car or a cat I saw never odd or even rats live on no evil star Your check should be case insensitive too. For example, "Bob" is a palindrome, despite the first B being capi..
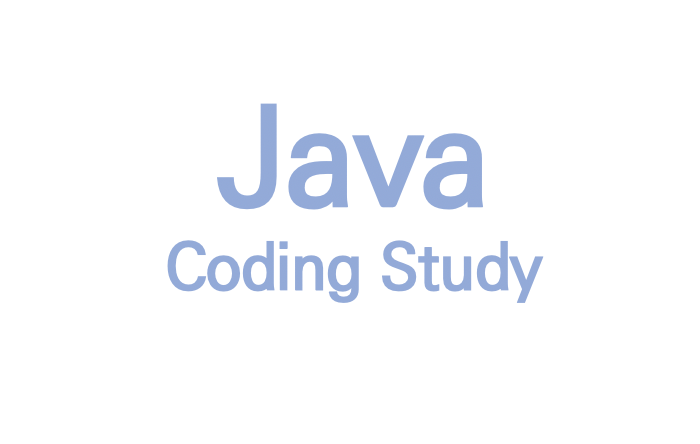
031 - Further For Loop Practice 7 (mIxEd CaSe)
Given the following inputs: String s; Write a for loop that will print out the string in alternating cases, with the first letter being lowercase. Keep in mind the following String methods: str.toUpperCase(); //make it uppercase str.toLowerCase(); //make it lowercase DO NOT USE .charAt()! .charAt returns a character, and you need a string in order to make it upper/lowercase. To get a letter at a..