auto-graded course
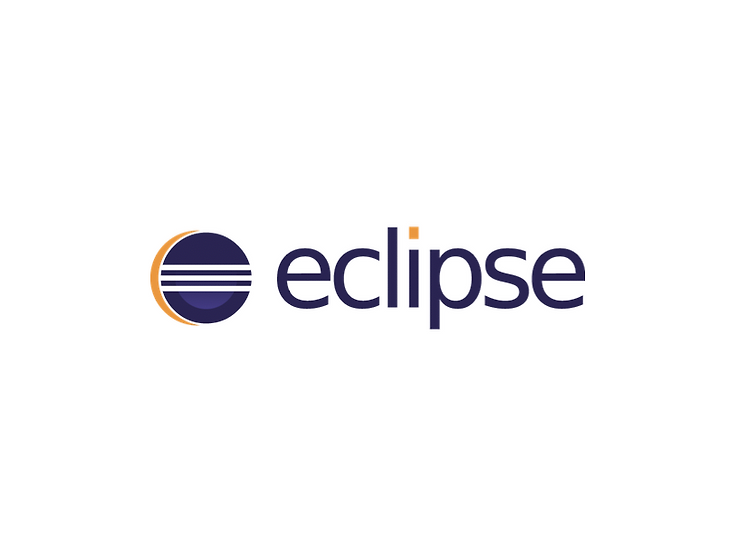
059 - Scope and Global Variables 2
Within the main class, create three class variables: A boolean variable called bool with a value of false A String variable called str with a value of "sup" An integer variable called i with a value of 10 Solution 1 2 3 4 5 6 7 8 9 10 class Main { boolean bool=false; String str="sup"; int i =10; public static void main(String[] args) { System.out.println("nothing to do here..."); } }
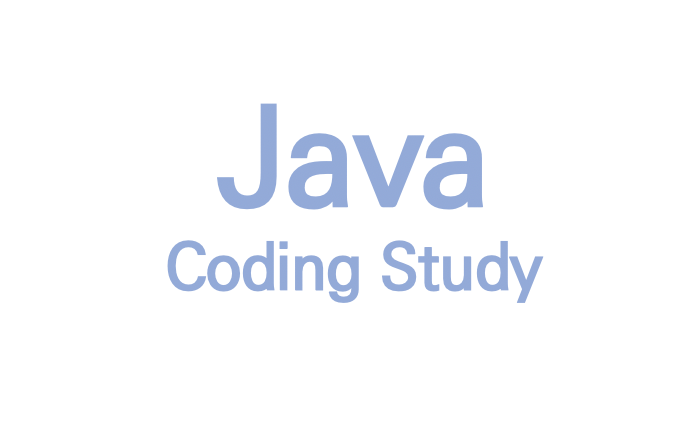
055 - Accumulator Method String Practice 3
Write a method header on line two with the following specs: Returns: a String Name: censorLetter Parameters: a String called s a char called ch Then complete the method by programming the following behavior Replace all instances of ch with a "*" within the String s. See below examples. Examples: censorLetter("computer science",'e') ==> "comput*r sci*nc*" censorLetter("trick or treat",'t') ==> "*..
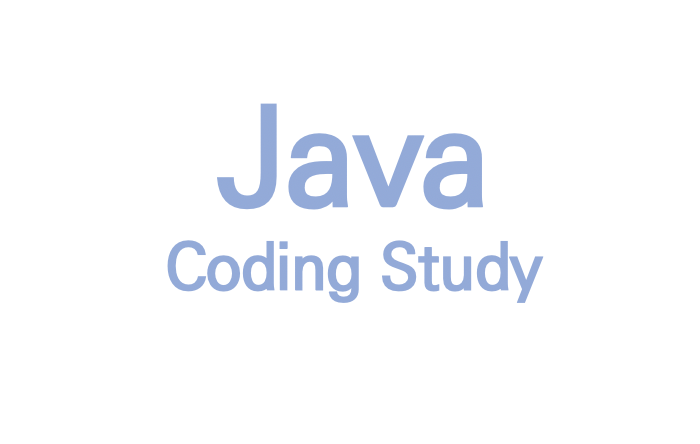
050 - Accumulator Method Practice 6
Write a method header on line two with the following specs: Returns: a String Name: keepVowels Parameters: a String called s Purpose: create a string that is composed of all the vowels in the string, in the same order that they appear. see below examples for clarity. Examples: keepVowels("hello") ==> "eo" keepVowels("how do i internets") ==> "ooiiee" Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 ..
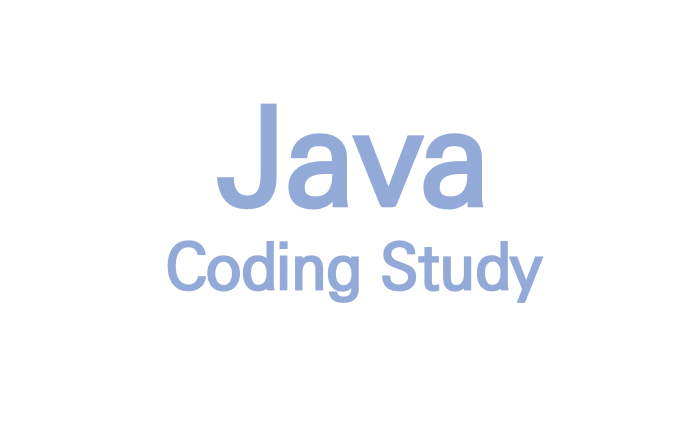
048 - Accumulator Method Practice 4
Write a method header on line two with the following specs: Returns: an integer Name: countA Parameters: a String called s Purpose: count the number of occurrences of 'a' or 'A' within s Examples: countA("aaa") ==> 3 countA("aaBBdf8k3AAadnklA") ==> 6 Hint: How do you write a for loop to loop through every letter of a string? You've done this multiple times already :) 1 2 3 4 5 6 7 8 9 10 11 12 1..
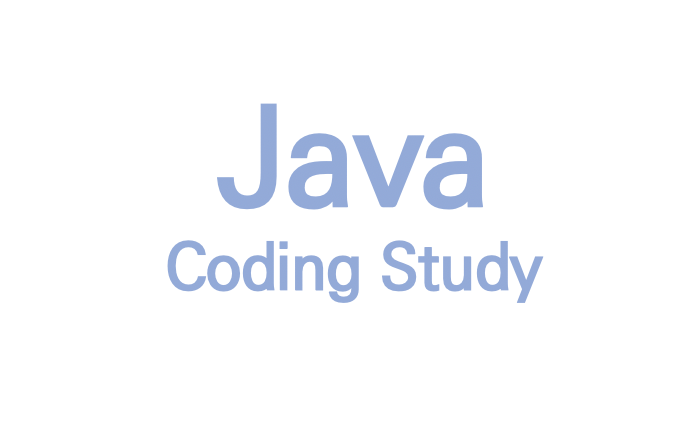
047- Accumulator Method Practice 3
Write a method header on line two with the following specs: Returns: an integer Name: sumFivesRange Parameters: an integer called "a" that represents the beginning of the range an integer called "b" that represents the end of the range Purpose: calculate the sum of the multiples of 5 within the range a to b inclusive (including b) Examples: sumFivesRange(5,15) ==> 30 sumFivesRange(11,28) ==> 60 ..
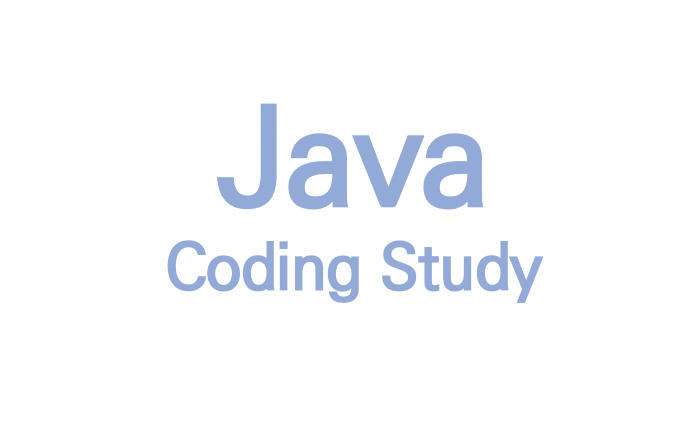
043 - Method Header Practice 8
Write a method header on line two with the following specs: Returns: a String Name: makeThreeSubstr Parameters: a String called "word" an integer called "startIndex" an integer called "endIndex" Then, starting on line 4, write code that will return 3x the substring (no spaces) from "startIndex" to "endIndex" Examples: makeThreeSubstr("hello",0,2) ==> "hehehe" makeThreeSubstr("shenanigans",3,7) =..
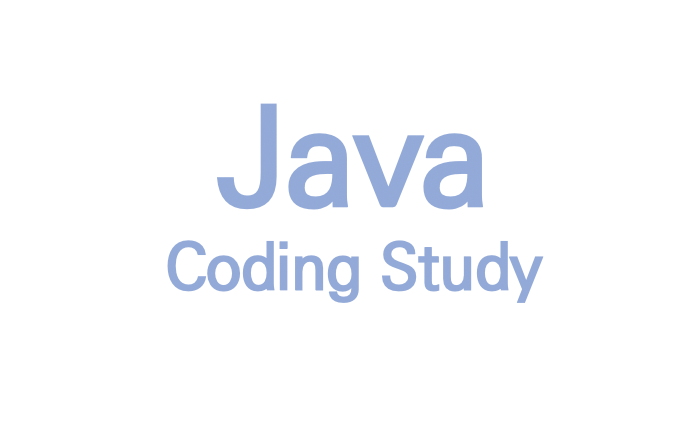
042 - Method Header Practice 7
Write a method header on line two with the following specs: Returns: a char Name: getChar Parameters: a String called "word" an integer called "index" Then, starting on line 4, write code that will return the character in "word" at the index "index" Examples: getChar("hello",1) ==> 'e' 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; class Main { public static char getChar(String word, int index) ..
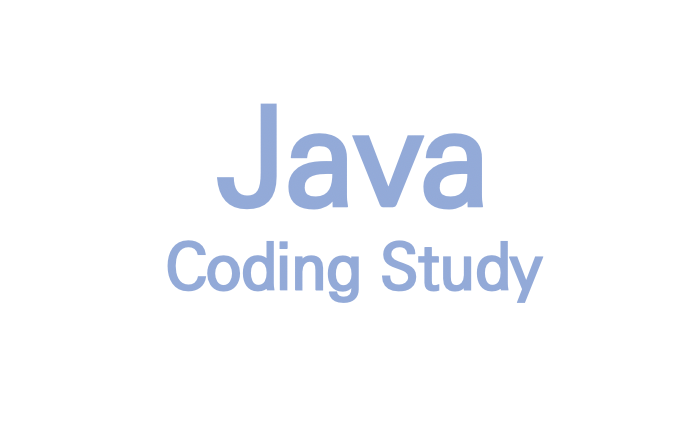
041 - Method Header Practice 6
Write a method header on line two with the following specs: Returns: a boolean Name: bothEven Parameters: an integer called "num1" an integer called "num2" Then, starting on line 4, write code that will return true if both num1 and num2 are even. Return false otherwise. Examples: bothEven(4,6) ==> true bothEven(3,4) ==> false bothEven(-1,1) ==> false 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.uti..
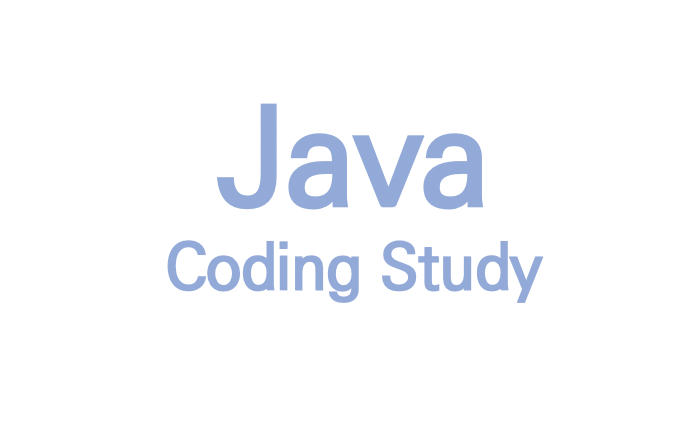
036 - Method Header Practice 1,2
036 - Method Header Practice 1 Write a method header on line two with the following specs: Returns: an integer Name: practiceOne Parameters: none You should not be writing code on any line other than #2 Solution 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; public class Main { public int practiceOne(){ { return 2; } } } 037 - Method Header Practice 2 Write a method header on line two with the f..
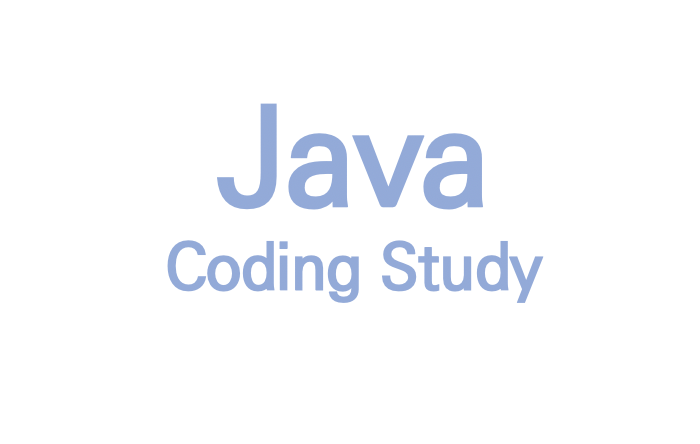
035 - Sum Odds in Range
You are given two inputs: int num1; int num2; You can assume that the following: num2 > num1 ==> TRUE You are to write a program that will calculate and print out the SUM OF ODD numbers in between num1 and num2 inclusive. Your output should all be on one line, separated by spaces. Sample input/output: #1: 3 #2: 11 Output: 35 #1: 4 #2: 20 Output: 96 #1: -2 #2: 6 Output: 8 Solution 1 2 3 4 5 6 7 8..
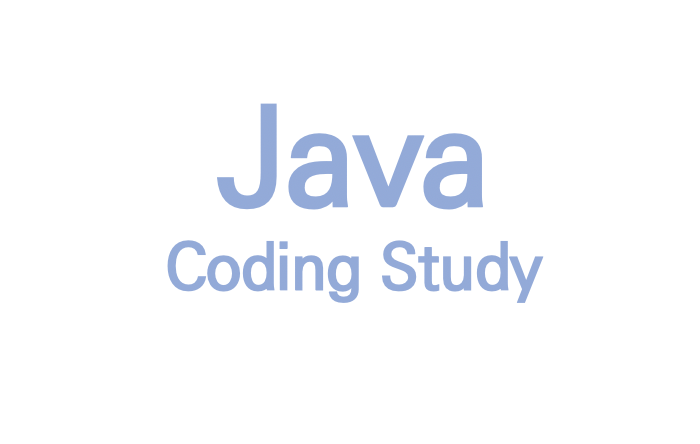
029 - Further For Loop Practice 5 (printing characters)
Given the following inputs: String s; Write a for loop that will print out each letter of the string s, separated by spaces, on the same line. Sample input/outputs: In: hello out: h e l l o In: covert out: c o v e r t In: blasphemy out: b l a s p h e m y Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Bakjoon { public static void main(String[] args) { Scanner inp = new ..
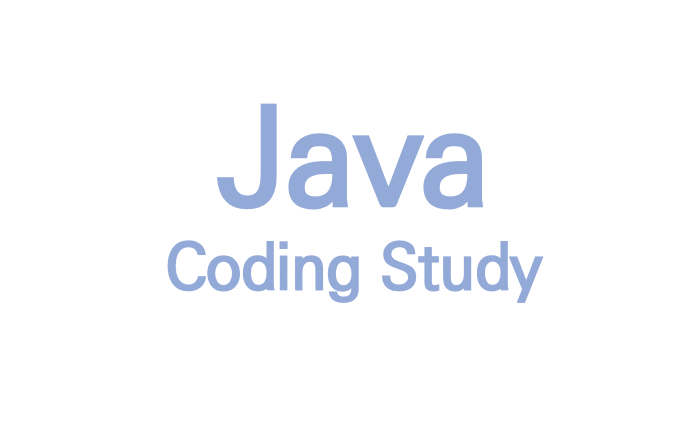
028 - Further For Loop Practice 4 (repeating X times)
Given the following inputs: int x; Write a for loop that will print out "AP CS A" x number of times, new line per print. Sample input/outputs: In: 3 AP CS A AP CS A AP CS A In: 5 AP CS A AP CS A AP CS A AP CS A AP CS A In: 1 AP CS A Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Bakjoon { public static void main(String[] args) { Scanner inp = new Scanner(System.in); Sy..
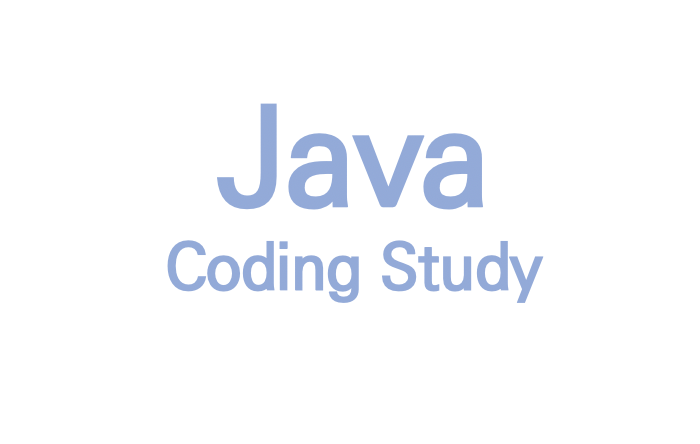
027 - Further For Loop Practice 3 (descending)
Given the following inputs: int x; Write a for loop that will print out a series of numbers starting at one less than x and ending at 0. Sample input/outputs: In: 7 out: 6 5 4 3 2 1 0 In: 12 out: 11 10 9 8 7 6 5 4 3 2 1 0 In: 20 out:19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1 0 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Main { public static void main(String[] a..
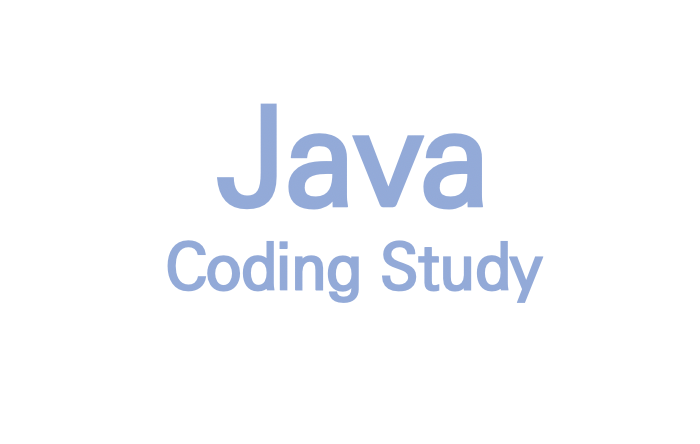
026 - Further For Loop Practice 2 (skipping by 3s)
Given the following inputs: int x; Write a for loop that will print out a series of numbers starting at 0 and ending before x, skipping 3 each time (excluding x). Sample input/outputs: In: 7 out: 0 3 6 In: 12 out: 0 3 6 9 In: 20 out: 0 3 6 9 12 15 18 HINT: You'll want to change the increment part of the for loop! 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 import java.util.*; class Bakjoon { public stat..