coding
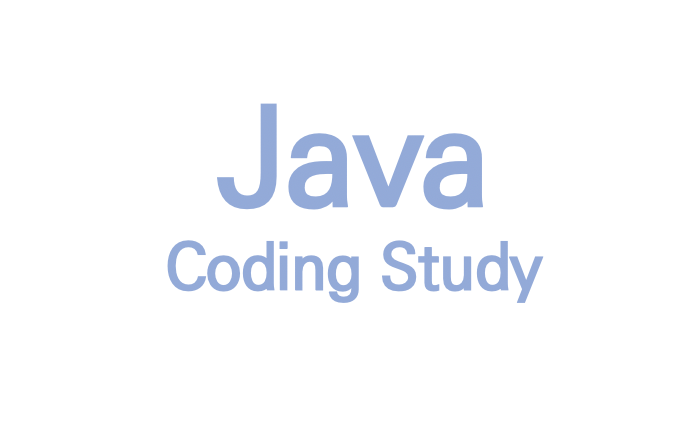
044 - Method Header Challenge 1 (Optional)
Write a method header on line two with the following specs: Returns: a String Name: mixString Parameters: a String called s1 a String called s2 Then, starting on line 4, write code that will return the strings combined, one letter at a time, starting with s1. See example below for an example. You can assume that s1 and s2 are equal lengths: s1 ==> "12345" s2 ==> "abcde" mixString(s1,s2) ==> "1a2..
![[백준/10990번] 별 찍기-15 [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FbcqZjl%2FbtqAixXCMNj%2F7BhrTewFNopYMCIFnRQXek%2Fimg.png)
[백준/10990번] 별 찍기-15 [Java]
문제 예제를 보고 규칙을 유추한 뒤에 별을 찍어 보세요. 입력 첫째 줄에 N(1 ≤ N ≤ 100)이 주어진다. 출력 첫째 줄부터 N번째 줄까지 차례대로 별을 출력한다. 풀이 앞에서 나오는 공간을 카운트 해주는 first_count, 그리고 별과 별 사이의 공간을 카운트 해주는 변수 last_count를 선언해주고 first_count는 개행될때마다 -1씩 해주고 last_count는 +1씩해주면서 카운트가 끝날때마다 별 하나를 찍어준다. ㅇㅅㅇ 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 import java.util.Scanner; class Bakjoon { public static void main(String[] args) throws Exce..
![[백준/ 16787번] マルバツスタンプ(JOI 2019) [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FpLRmG%2FbtqAjMlR9dc%2F8uSxkDwk82XyYfWms0qHfk%2Fimg.png)
[백준/ 16787번] マルバツスタンプ(JOI 2019) [Java]
Problem JOI 君はマルスタンプ,バツスタンプ,マルバツスタンプの3種類のスタンプをそれぞれ 0 個以上持っている.これらはマルやバツのマークを紙に印字することができるスタンプである. マルスタンプを使うとマルが 1 つ印字され,バツスタンプを使うとバツが 1 つ印字される.マルバツスタンプを使うとマルとバツが横一列に 1 つずつ印字され,スタンプの向きを変えることで,マルの右にバツが来るようにも,バツの右にマルが来るようにも印字できる. JOI 君は,持っているスタンプをそれぞれちょうど 1 回ずつ適当な順番で使い,紙に横一列にマルとバツを印字した.印字されたマルとバツの列は文字列 S で表される.S は O と X から構成された長さ N の文字列であり,S_i = O ならば JOI 君が印字したマークのうち左から i 番目のものがマルであることを表し,S_i = X な..
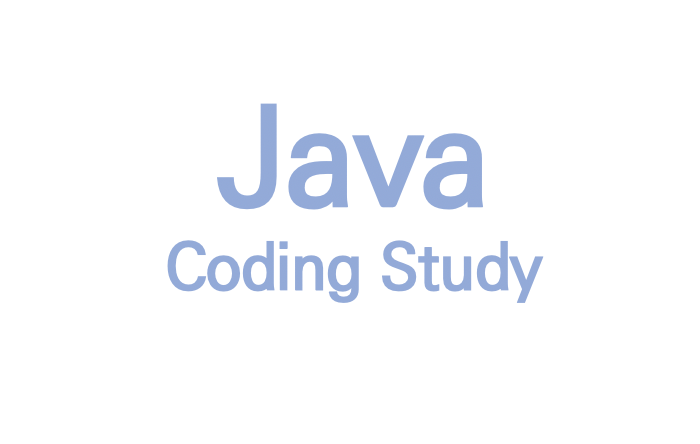
031 - Further For Loop Practice 7 (mIxEd CaSe)
Given the following inputs: String s; Write a for loop that will print out the string in alternating cases, with the first letter being lowercase. Keep in mind the following String methods: str.toUpperCase(); //make it uppercase str.toLowerCase(); //make it lowercase DO NOT USE .charAt()! .charAt returns a character, and you need a string in order to make it upper/lowercase. To get a letter at a..
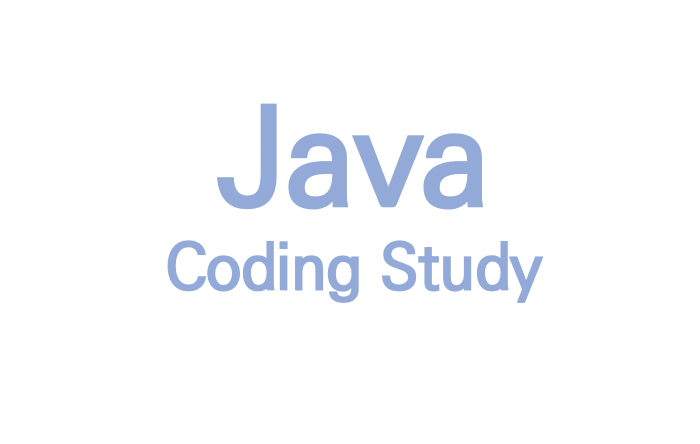
030 - Further For Loop Practice 6 (reverse string)
Given the following inputs: String s; Write a for loop that will print out the reverse of the string. Sample input/outputs: In: manhattan out: nattahnam In: processor out: rossecorp In: racecar out: racecar Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Bakjoon { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.out.print("In:"); Str..
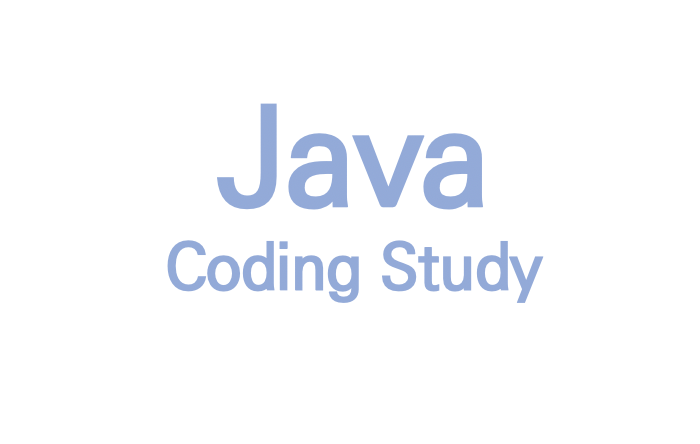
029 - Further For Loop Practice 5 (printing characters)
Given the following inputs: String s; Write a for loop that will print out each letter of the string s, separated by spaces, on the same line. Sample input/outputs: In: hello out: h e l l o In: covert out: c o v e r t In: blasphemy out: b l a s p h e m y Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Bakjoon { public static void main(String[] args) { Scanner inp = new ..
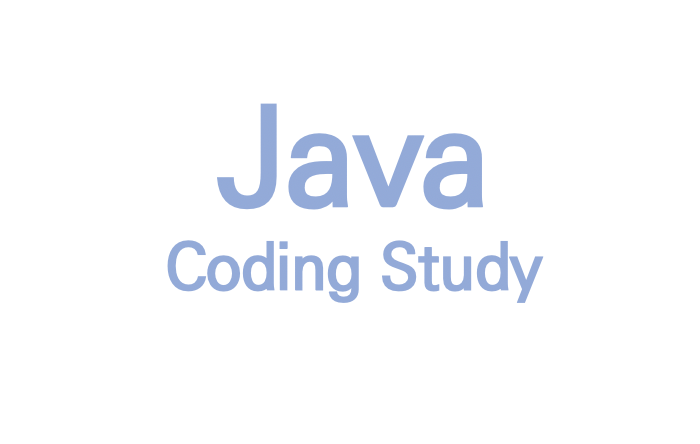
027 - Further For Loop Practice 3 (descending)
Given the following inputs: int x; Write a for loop that will print out a series of numbers starting at one less than x and ending at 0. Sample input/outputs: In: 7 out: 6 5 4 3 2 1 0 In: 12 out: 11 10 9 8 7 6 5 4 3 2 1 0 In: 20 out:19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1 0 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Main { public static void main(String[] a..
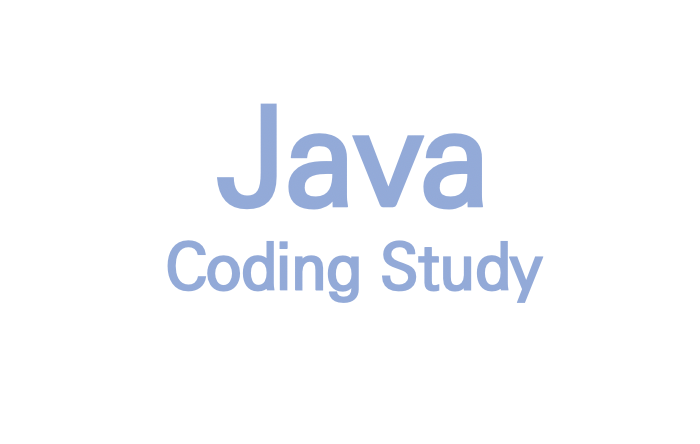
026 - Further For Loop Practice 2 (skipping by 3s)
Given the following inputs: int x; Write a for loop that will print out a series of numbers starting at 0 and ending before x, skipping 3 each time (excluding x). Sample input/outputs: In: 7 out: 0 3 6 In: 12 out: 0 3 6 9 In: 20 out: 0 3 6 9 12 15 18 HINT: You'll want to change the increment part of the for loop! 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 import java.util.*; class Bakjoon { public stat..
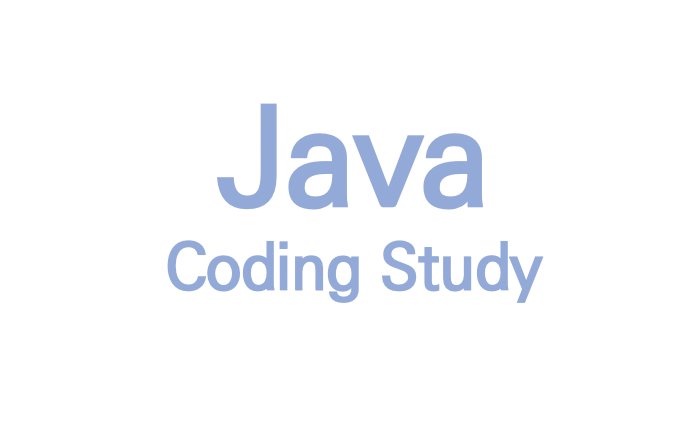
025 - Further For Loop Practice 1 (ascending)
Given the following inputs: int x; Write a for loop that will print out a series of numbers starting at 0 and ending at the x, exclusive. Sample input/outputs: In: 3 out: 0 1 2 In: 8 out: 0 1 2 3 4 5 6 7 In: 5 out: 0 1 2 3 4 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 import java.util.*; class Main { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.out.print..
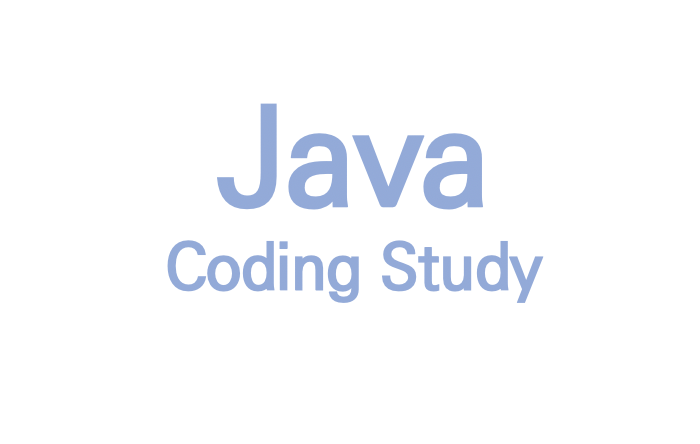
024 - For Loop 6 (optional)
Using a for loop, determine whether the integer variable max is prime or not. If the number is prime, print out "prime". If it's not, print out "not prime". Keep in mind that 1 is NOT a prime number. You can use the following to find the square root of a number (you may or may not use this): Math.sqrt(num) Sample input/outputs: In: 100 out: not prime In: 17 out: prime In: 9 out: not prime In: 1 ..
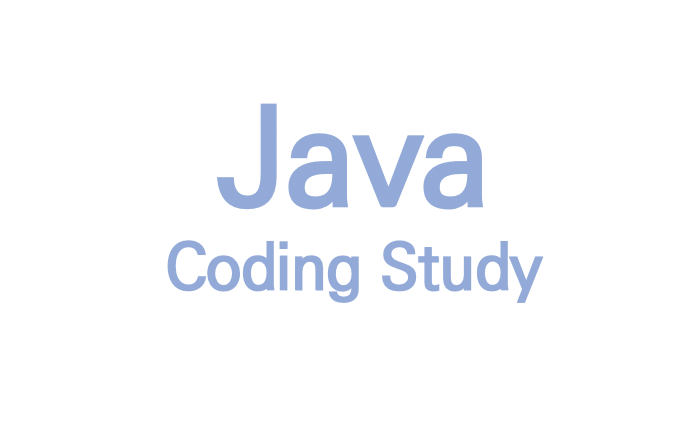
020 - For Loops 2
Write a for loop that will print out the numbers starting at 1 and ending at twice the end number exclusive. Each number should be on the same line, separated by a space. Sample inputs/outputs: In: 5 0 1 2 3 4 5 6 7 8 9 In: 10 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 In: -5 (no output) 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 import java.util.Scanner; class Main { public static void..
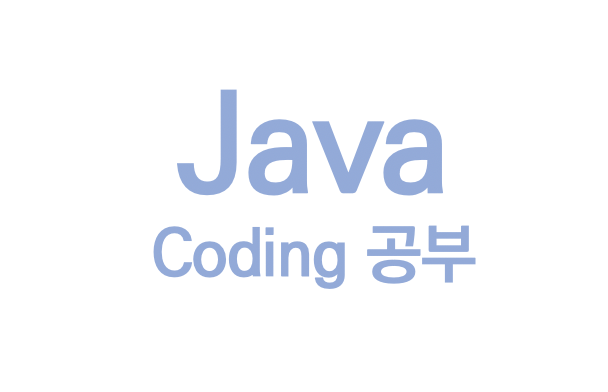
019 - For Loops 1
Inputs: int end; Write a for loop that will print out the numbers starting at 1 and ending at the input inclusive. The numbers printed should all be on the same line separated by a space. Sample inputs/outputs: In: 5 1 2 3 4 5 In: 10 1 2 3 4 5 6 7 8 9 10 In: -5 (no output) 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 import java.util.Scanner; class Main { public static void main(String[] args) {..
![[Programmers] 콜라츠 추측 (Collatz conjecture) [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2Fcqj3Hi%2Fbtqz4L28RmE%2FAfhZun8l5ufAYaMunL8HdK%2Fimg.png)
[Programmers] 콜라츠 추측 (Collatz conjecture) [Java]
문제 설명 1937년 Collatz란 사람에 의해 제기된 이 추측은, 주어진 수가 1이 될때까지 다음 작업을 반복하면, 모든 수를 1로 만들 수 있다는 추측입니다. 작업은 다음과 같습니다. 1-1. 입력된 수가 짝수라면 2로 나눕니다. 1-2. 입력된 수가 홀수라면 3을 곱하고 1을 더합니다. 2. 결과로 나온 수에 같은 작업을 1이 될 때까지 반복합니다. 예를 들어, 입력된 수가 6이라면 6→3→10→5→16→8→4→2→1 이 되어 총 8번 만에 1이 됩니다. 위 작업을 몇 번이나 반복해야하는지 반환하는 함수, solution을 완성해 주세요. 단, 작업을 500번을 반복해도 1이 되지 않는다면 –1을 반환해 주세요. 제한 사항 입력된 수, num은 1 이상 8000000 미만인 정수입니다. 입출력 예..
![[Programmers] 서울에서 김서방 찾기 [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FcJx0dv%2Fbtqz5bfQWte%2Fxcjk68AIkteKWn0315oU0K%2Fimg.png)
[Programmers] 서울에서 김서방 찾기 [Java]
문제 설명 String형 배열 seoul의 element중 Kim의 위치 x를 찾아, 김서방은 x에 있다는 String을 반환하는 함수, solution을 완성하세요. seoul에 Kim은 오직 한 번만 나타나며 잘못된 값이 입력되는 경우는 없습니다. 제한 사항 seoul은 길이 1 이상, 1000 이하인 배열입니다. seoul의 원소는 길이 1 이상, 20 이하인 문자열입니다. Kim은 반드시 seoul 안에 포함되어 있습니다. 입출력 예 seoul return [Jane, Kim] 김서방은 1에 있다 풀이 1 2 3 4 5 6 7 8 9 10 11 class Solution { String answer = ""; public String solution(String[] seoul) { for(int ..
![[Programmers] 짝수와 홀수 [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FbPX6M2%2Fbtqz3A1Y8Xo%2Ffr8otU1qH5RdeToSrSgPa0%2Fimg.png)
[Programmers] 짝수와 홀수 [Java]
문제 설명 정수 num이 짝수일 경우 Even을 반환하고 홀수인 경우 Odd를 반환하는 함수, solution을 완성해주세요. 제한 조건 num은 int 범위의 정수입니다. 0은 짝수입니다. 입출력 예 num return 3 Odd 4 Even 풀이 1 2 3 4 5 6 class Solution { public String solution(int num) { String answer = num%2==0? "Even":"Odd"; return answer; } } 정수형 num을 2로 나누어서 나머지가 0 -> Even 나머지가 0이 아니다 ->Odd ㅇㅅㅇ 아래에서 문제를 확인하고 한번씩 풀어보세요~!! https://programmers.co.kr/learn/courses/30/lessons/1293..
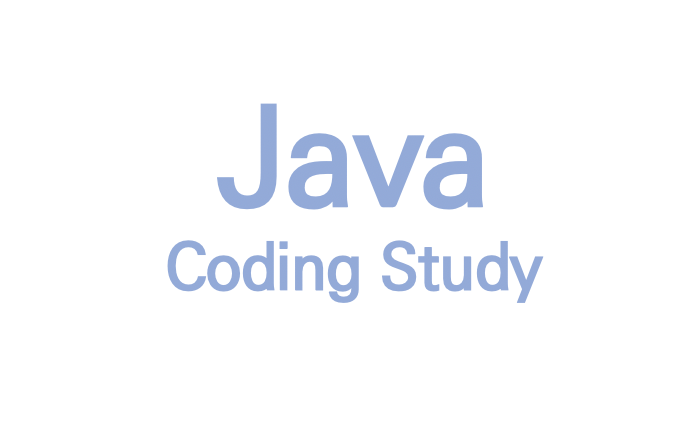
017 - Conditional Statement Practice 3
The variable "name" holds a String user input Write a conditional statement starting on line 9 that does the following: If name is equal to "Chen", print "teacher" For any other input, print "student" Examples: In: Chen teacher In: Faa student 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.util.*; class Main { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.out.p..
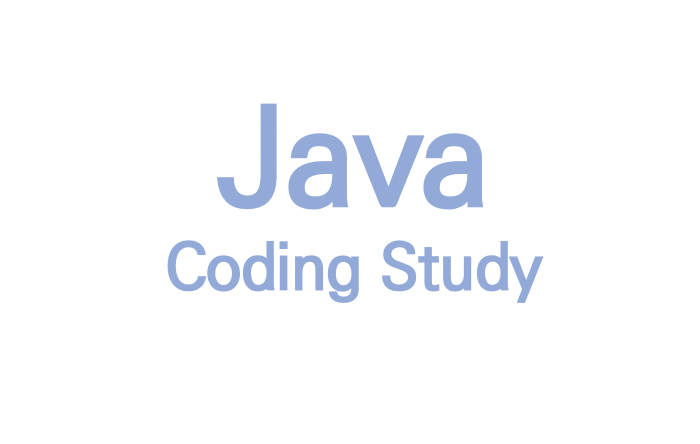
016 - Conditional Statement Practice 2
The variable "num" holds an integer user input Write a conditional statement starting on line 9 that does the following: If num is even (divisible by 2), print "__ is even" If num is odd, print "__ is odd" Examples: In: 23 23 is odd In: 36 36 is even 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; class Main { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.o..
![[Bakjoon/3052번] 나머지 [Java]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FbYGEaH%2Fbtqz3B6TTeH%2F0bK6S2HehgWTuH1QM6Kmk0%2Fimg.png)
[Bakjoon/3052번] 나머지 [Java]
아래포스팅을 통해 문제를 볼 수 있습니다!! https://www.acmicpc.net/problem/1546 1546번: 평균 첫째 줄에 시험 본 과목의 개수 N이 주어진다. 이 값은 1000보다 작거나 같다. 둘째 줄에 세준이의 현재 성적이 주어진다. 이 값은 100보다 작거나 같은 음이 아닌 정수이고, 적어도 하나의 값은 0보다 크다. www.acmicpc.net 문제 두 자연수 A와 B가 있을 때, A%B는 A를 B로 나눈 나머지 이다. 예를 들어, 7, 14, 27, 38을 3으로 나눈 나머지는 1, 2, 0, 2이다. 수 10개를 입력받은 뒤, 이를 42로 나눈 나머지를 구한다. 그 다음 서로 다른 값이 몇 개 있는지 출력하는 프로그램을 작성하시오. 입력 첫째 줄부터 열번째 줄 까지 숫자가 한..
![[Programmers] 이름이 있는 동물의 아이디 [mySQL]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2Fkx1Vm%2Fbtqz3AePtMn%2FQHlfXiW5MtL7qGWbFJgrEK%2Fimg.png)
[Programmers] 이름이 있는 동물의 아이디 [mySQL]
본 문제는 Kaggle의 Austin Animal Center Shelter Intakes and Outcomes에서 제공하는 데이터를 사용하였으며 ODbL의 적용을 받습니다. 문제 설명 ANIMAL_INS 테이블은 동물 보호소에 들어온 동물의 정보를 담은 테이블입니다. ANIMAL_INS 테이블 구조는 다음과 같으며, ANIMAL_ID, ANIMAL_TYPE, DATETIME, INTAKE_CONDITION, NAME, SEX_UPON_INTAKE는 각각 동물의 아이디, 생물 종, 보호 시작일, 보호 시작 시 상태, 이름, 성별 및 중성화 여부를 나타냅니다. NAME TYPE NULLABLE ANIMAL_ID VARCHAR(N) FALSE ANIMAL_TYPE VARCHAR(N) FALSE DATETI..
![[Programmers] 아픈 동물 찾기 [mySQL]](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FbiSUhu%2Fbtqz3ntG36E%2FXZPK4Tf9qKPJloHKj2q61k%2Fimg.png)
[Programmers] 아픈 동물 찾기 [mySQL]
본 문제는 Kaggle의 Austin Animal Center Shelter Intakes and Outcomes에서 제공하는 데이터를 사용하였으며 ODbL의 적용을 받습니다. 문제 설명 ANIMAL_INS 테이블은 동물 보호소에 들어온 동물의 정보를 담은 테이블입니다. ANIMAL_INS 테이블 구조는 다음과 같으며, ANIMAL_ID, ANIMAL_TYPE, DATETIME, INTAKE_CONDITION, NAME, SEX_UPON_INTAKE는 각각 동물의 아이디, 생물 종, 보호 시작일, 보호 시작 시 상태, 이름, 성별 및 중성화 여부를 나타냅니다. NAME TYPE NULLABLE ANIMAL_ID VARCHAR(N) FALSE ANIMAL_TYPE VARCHAR(N) FALSE DATETI..