레플릿
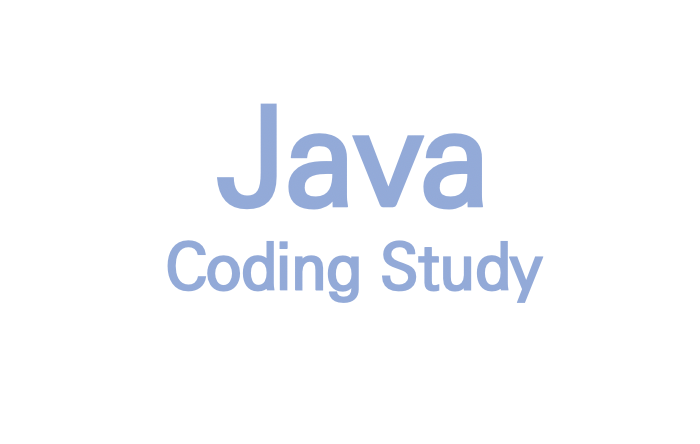
058 - Scope and Global Variables 1
Fix the following code so it produces the following output: one word Note: You should achieve this WITHOUT changing any print statements. Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 import java.util.*; class Main { public static void main(String[] args) { String output =""; String text = "hello"; if (text.indexOf(" ") == -1) //if a space doesn't exist { output = "one word"; } else {..
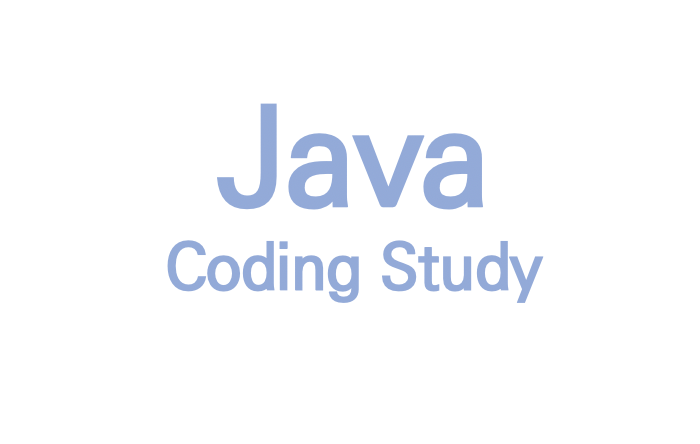
056 - Accumulator Method String Practice 4
Write a method header on line two with the following specs: Returns: a String Name: surround Parameters: a String called s a char called search_term Then complete the method by programming the following behavior Return a new String built from s that has every instance of the search term surrounded by parentheses See below examples. Examples: surround("abcabcabc",'c') ==> "ab(c)ab(c)ab(c)" surrou..
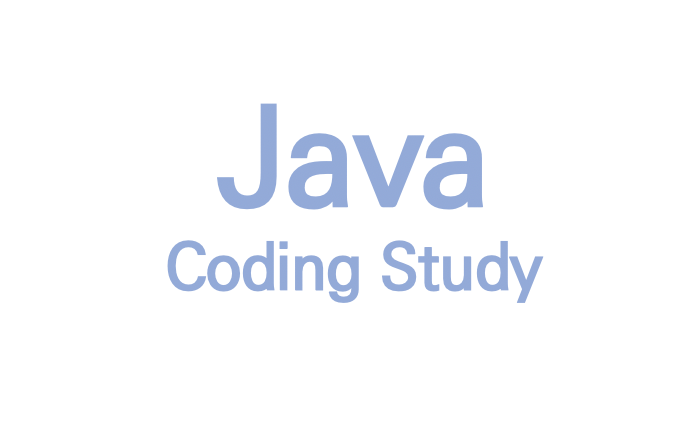
055 - Accumulator Method String Practice 3
Write a method header on line two with the following specs: Returns: a String Name: censorLetter Parameters: a String called s a char called ch Then complete the method by programming the following behavior Replace all instances of ch with a "*" within the String s. See below examples. Examples: censorLetter("computer science",'e') ==> "comput*r sci*nc*" censorLetter("trick or treat",'t') ==> "*..
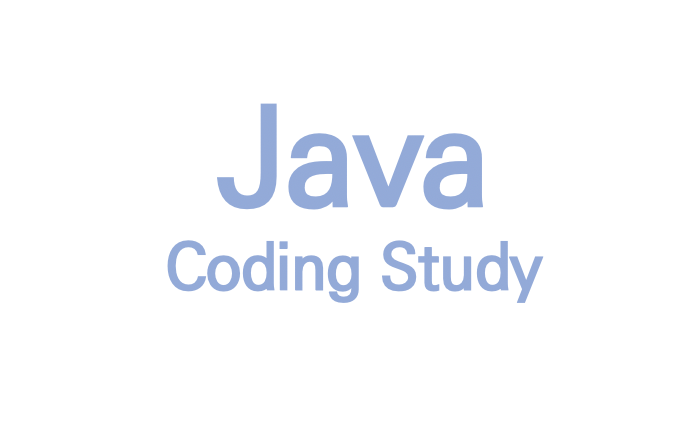
051 - Accumulator Method Challenge 1 (optional)
Write a method header on line two with the following specs: Returns: an integer Name: countString Parameters: a String called str a String called toFind Purpose: Count the number of occurrences of toFind within str. See below examples. Examples: countString("crazy crayfish crushing craniums", "cra") ==> 3 countString("sometimes solutions dont come on time", "me") ==> 4 Solution 1 2 3 4 5 6 7 8 9..
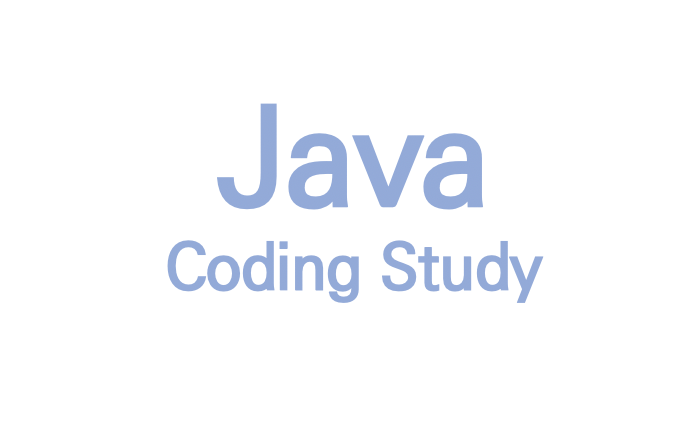
049 - Accumulator Method Practice 5
Write a method header on line two with the following specs: Returns: an integer Name: countVowels Parameters: a String called s Purpose: count the number of vowels in the string s. Assume s is all lowercase. Examples: countVowels("obama") ==> 3 countVowels("happy friday! i love weekends") ==> 9 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 class Main { public static int..
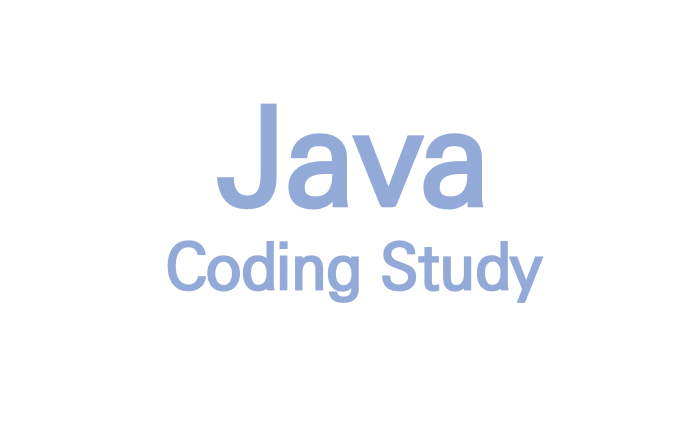
048 - Accumulator Method Practice 4
Write a method header on line two with the following specs: Returns: an integer Name: countA Parameters: a String called s Purpose: count the number of occurrences of 'a' or 'A' within s Examples: countA("aaa") ==> 3 countA("aaBBdf8k3AAadnklA") ==> 6 Hint: How do you write a for loop to loop through every letter of a string? You've done this multiple times already :) 1 2 3 4 5 6 7 8 9 10 11 12 1..
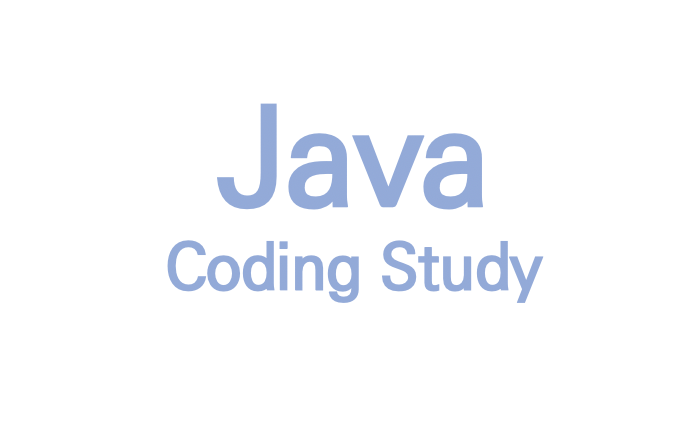
044 - Method Header Challenge 1 (Optional)
Write a method header on line two with the following specs: Returns: a String Name: mixString Parameters: a String called s1 a String called s2 Then, starting on line 4, write code that will return the strings combined, one letter at a time, starting with s1. See example below for an example. You can assume that s1 and s2 are equal lengths: s1 ==> "12345" s2 ==> "abcde" mixString(s1,s2) ==> "1a2..
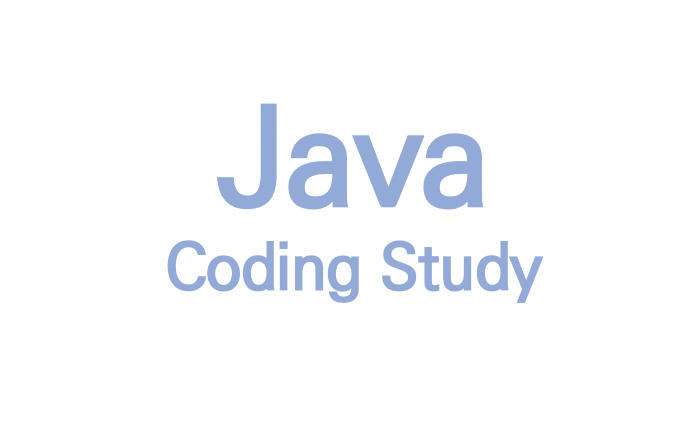
043 - Method Header Practice 8
Write a method header on line two with the following specs: Returns: a String Name: makeThreeSubstr Parameters: a String called "word" an integer called "startIndex" an integer called "endIndex" Then, starting on line 4, write code that will return 3x the substring (no spaces) from "startIndex" to "endIndex" Examples: makeThreeSubstr("hello",0,2) ==> "hehehe" makeThreeSubstr("shenanigans",3,7) =..
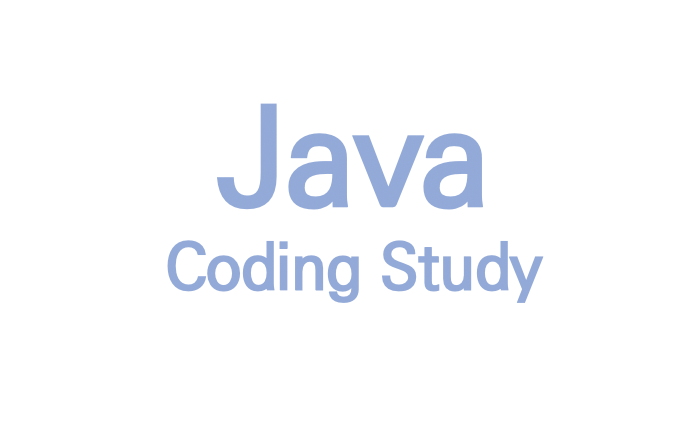
042 - Method Header Practice 7
Write a method header on line two with the following specs: Returns: a char Name: getChar Parameters: a String called "word" an integer called "index" Then, starting on line 4, write code that will return the character in "word" at the index "index" Examples: getChar("hello",1) ==> 'e' 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; class Main { public static char getChar(String word, int index) ..
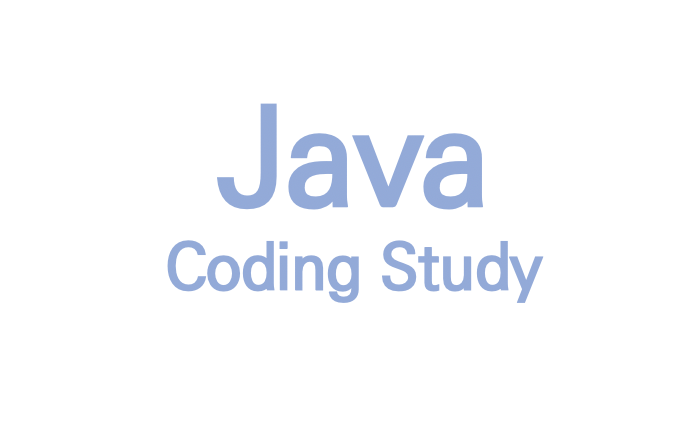
034 - Big Number Program (From Decoding)
You are given two inputs: int num1; int num2; You can assume that the following: num2 > num1 ==> TRUE You are to write a program that will print out all the ODD numbers in between num1 and num2 inclusive. Your output should all be on one line, separated by spaces. Sample input/output: #1: 3 #2: 11 3 5 7 9 11 #1: 4 #2: 20 5 7 9 11 13 15 17 19 #1: -2 #2: 6 -1 1 3 5 Solution 1 2 3 4 5 6 7 8 9 10 11..
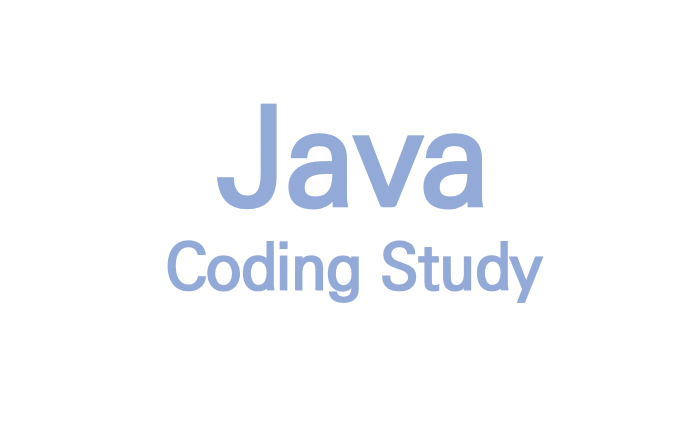
018 - Conditional Statement Practice 4
For you to do: Given a string variable "word", do the following tests If the word ends in "y", print "-ies" If the word ends in "ey", print "-eys" If the word ends in "ife", print "-ives" If none of the above is true, print "-s" No more than one should be printed. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 import java.util.*; class Main { public static void ..
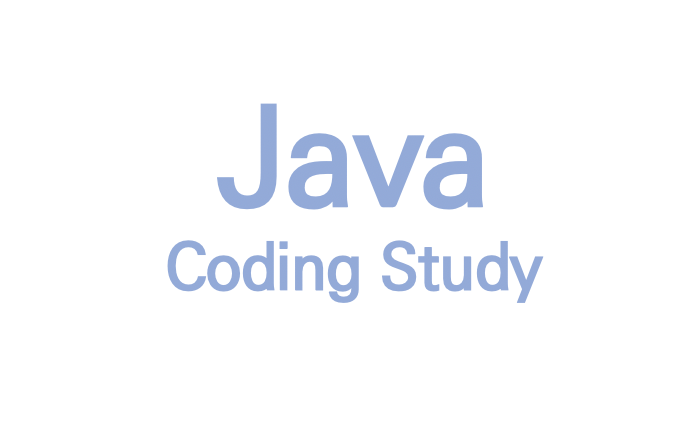
017 - Conditional Statement Practice 3
The variable "name" holds a String user input Write a conditional statement starting on line 9 that does the following: If name is equal to "Chen", print "teacher" For any other input, print "student" Examples: In: Chen teacher In: Faa student 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.util.*; class Main { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.out.p..
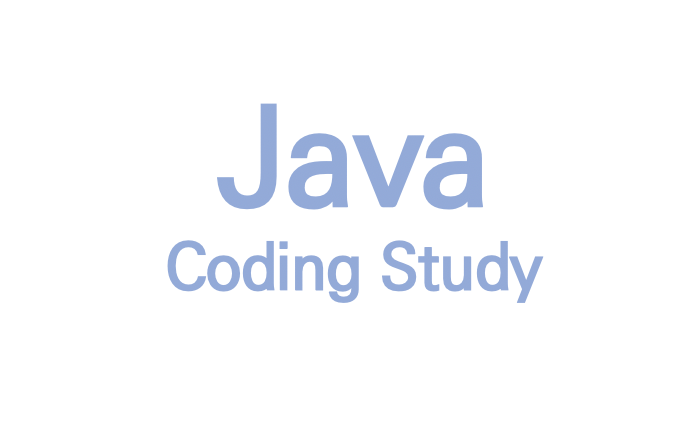
015 - Conditional Statement Practice 1
The variable "num" holds an integer user input Write a conditional statement starting on line 9 that does the following: If num is positive, print "__ is positive" If num is negative, print "__ is negative" Examples: In: 5 5 is positive In: -2 -2 is negative In: 0 (no output for zero) import java.util.*; class Main { public static void main(String[] args) { Scanner inp = new Scanner(System.in); ..
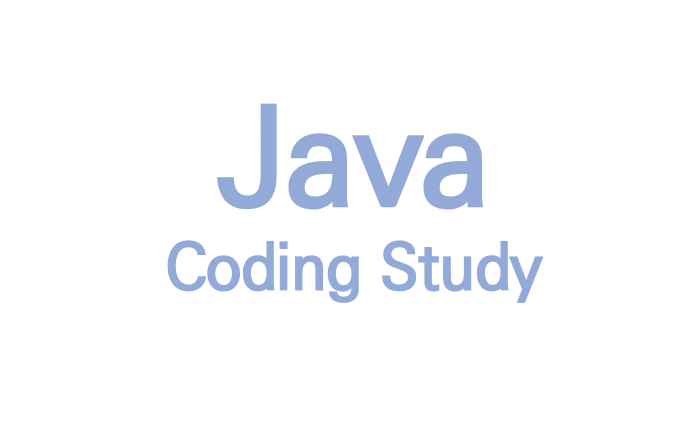
011 - String Methods Practice 2
Given three variables: String str int start int end Print out the following string: The substring of (str) from (start) to (end) is (substring from start to end, inclusive) Sample output: In: lolwut Start: 2 End: 4 The substring of lolwut from 2 to 4 inclusive is lwu PLEASE NOTE that we are counting the end index in our output! lolwut 012345 import java.util.Scanner; class Main { public static v..
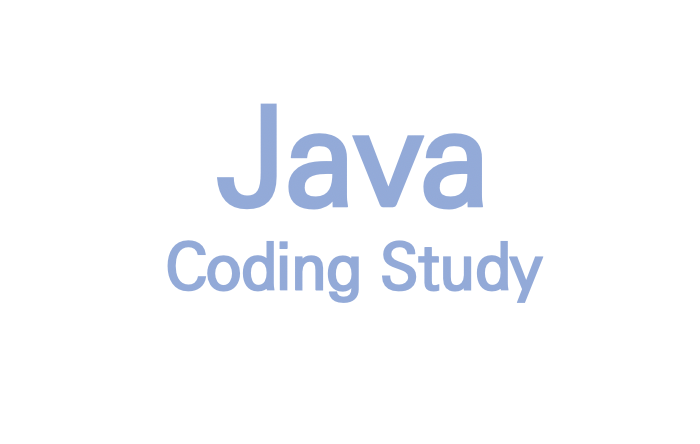
010 - String Methods Practice 1
Given a String (already declared for you as str), do the following: Print out the following: "The first 3 letters of ___ is ___" For example, if the input is "banana", your output should be "The first 3 letters of banana is ban" import java.util.Scanner; class Main { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.out.print("in:"); String str = inp.nextLine(..
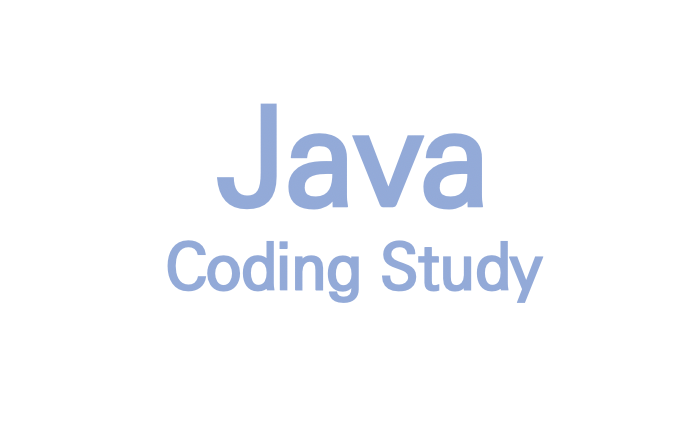
009 - String Methods - substring
For you to do: (all output should be one per line) On line 6, write a print statement that prints out a substring of str starting at index 5 and going to the end On line 7, write a print statement that prints out a substring of str starting at index 7 and ending at index 10 On line 10, fill in the () in substring so that it prints "harambe" On line 11, fill in the () in substring so that it prin..
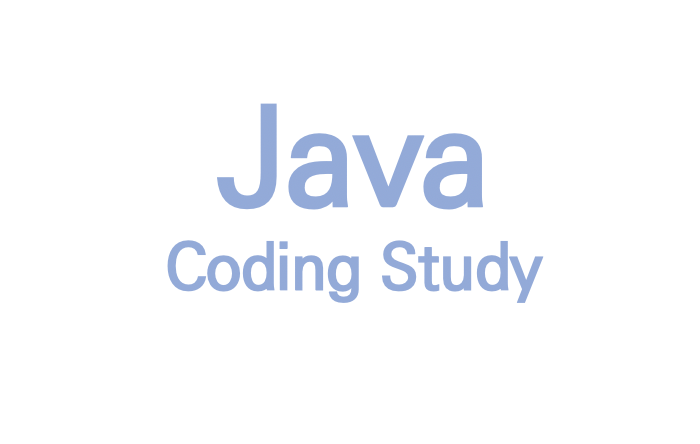
008 - String Methods - indexOf
For you to do: (the output should be one per line) Print out the position of the first occurrence of "c" Print out the position of the first occurrence of " " Print out the position of the first occurrence of the variable target1 Print out the position of the first occurrence of the variable target2 class Main { public static void main(String[] args) { String str = "abracadabra alakazam"; String..
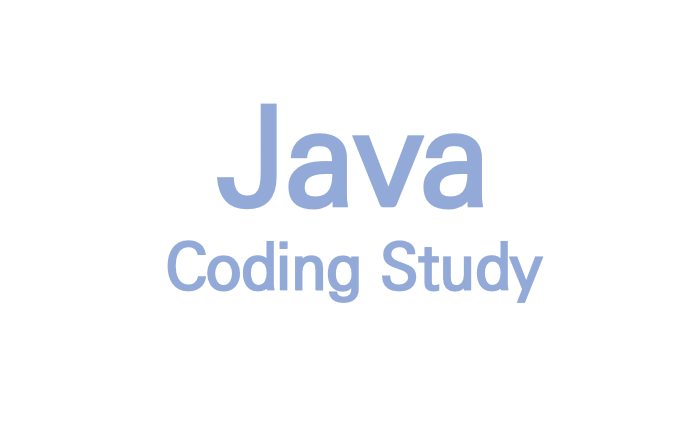
007 - String Methods - charAt
For you to do: ALL print statements in this exercise should be on one line, no newlines. Print out the character in the 5th index of the String str Print out the character in the 8th index of the String str Print out the character in the 1st index of the String str Print out the index in the i-th index of the String str (i is a variable already declared for you) class Main { public static void m..
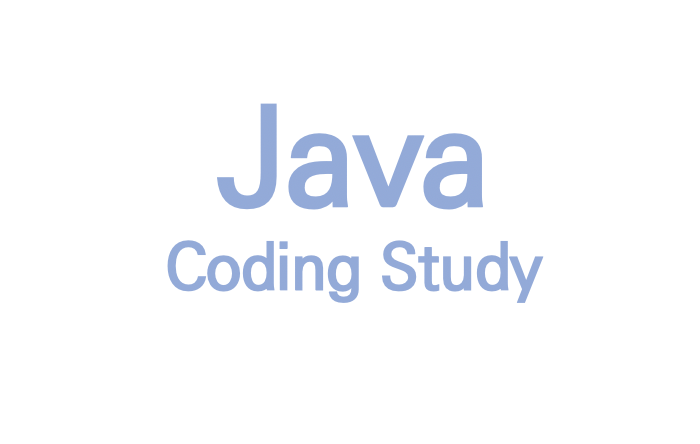
006 - String Methods - Length
For you to do: Create a String named "name" and assign the value "Timmy" to it Print out the length of the string variable 'name' class Main { public static void main(String[] args) { String name="Timmy"; System.out.println(name.length()); } }
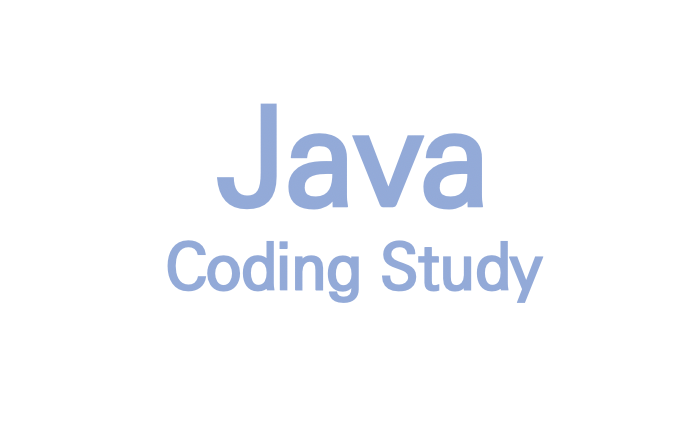
005 - Creating Variables and Printing 5
Creating Variables and Printing 5 For you to do: Create a String variable called "name" and set it to "Chen" Create an integer variable called "age" and set it to 50 Create an integer variable called "iq" and set it to the value of age (do NOT use ' = 50') Print the value of name Print the value of age without skipping a new line Print the value of iq class Main { public static void main(String[..