auto graded course
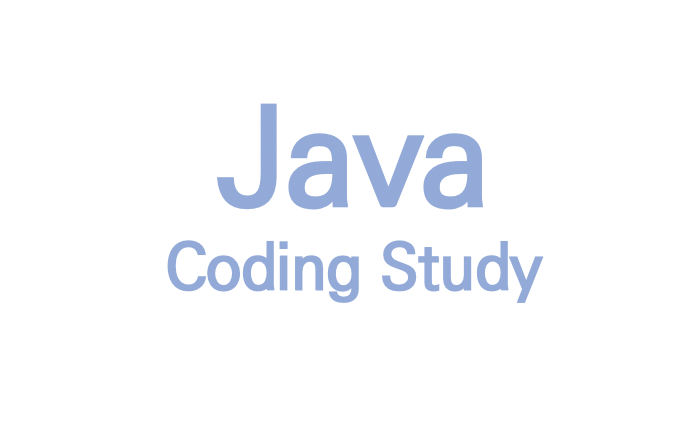
057 - Accumulator Method String Challenge 1 (optional)
Write a method header on line two with the following specs: Returns: a String Name: surroundStr Parameters: a String called s a String called search_term Then complete the method by programming the following behavior Return a new String built from s that has every instance of the search term surrounded by parentheses See below examples. Examples: surroundStr("abcabcabc","abc") ==> "(abc)(abc)(ab..
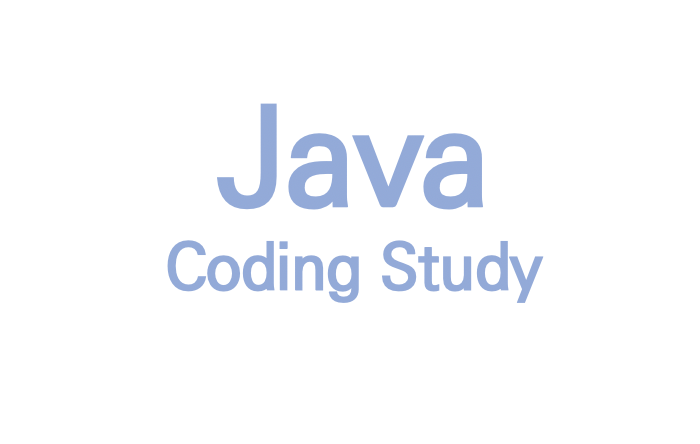
056 - Accumulator Method String Practice 4
Write a method header on line two with the following specs: Returns: a String Name: surround Parameters: a String called s a char called search_term Then complete the method by programming the following behavior Return a new String built from s that has every instance of the search term surrounded by parentheses See below examples. Examples: surround("abcabcabc",'c') ==> "ab(c)ab(c)ab(c)" surrou..
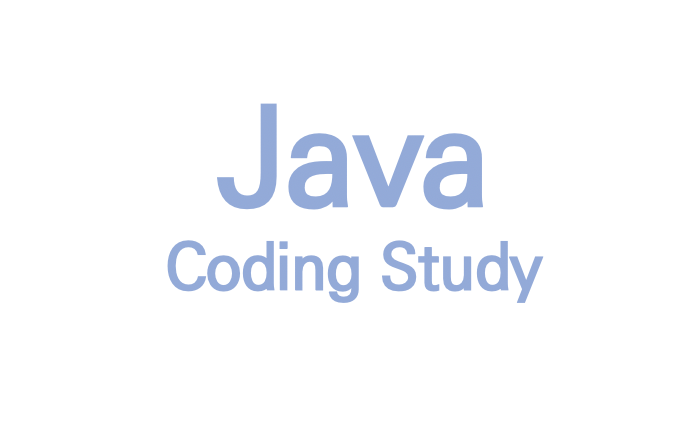
054 - Accumulator Method String Practice 2
Write a method header on line two with the following specs: Returns: a String Name: thirdLetter Parameters: a String called s Then complete the method by programming the following behavior Returns every 3rd letter of the String s, starting the first letter. See below examples. Examples: thirdLetter("hello there") ==> "hltr" thirdLetter("technology") ==> "thly" Solution 1 2 3 4 5 6 7 8 9 10 11 12..
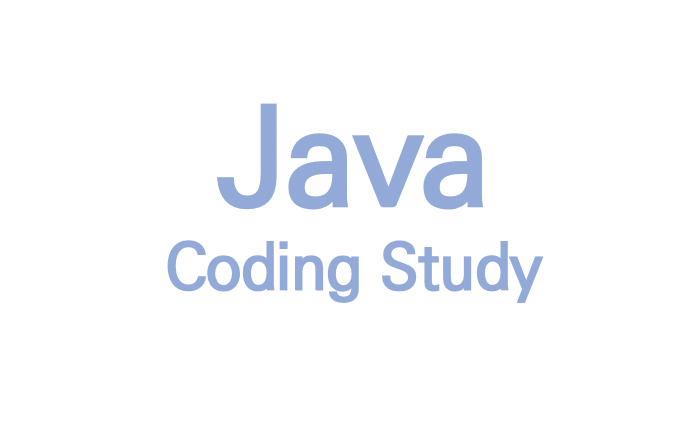
053 - Accumulator Method String Practice 1
Write a method header on line two with the following specs: Returns: a String Name: spaceOut Parameters: a String called s Then complete the method by programming the following behavior Insert spaces after every character in the String s, then return the new string. See below examples (note the space at the end as well). Examples: spaceOut("hello") ==> "h e l l o " spaceOut("technology") ==> "t ..
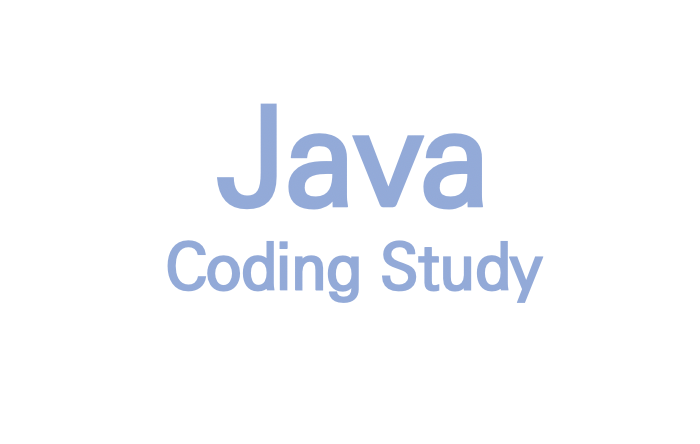
052 - Accumulator Method Challenge 2 (optional)
Write a method header on line two with the following specs: Returns: a String Name: alphabetical Parameters: a String called str Purpose: Return a string that is composed of each letter as long as the letter is later on in the alphabet than its previous one. You can assume actual parameters are lowercase. See below examples. Additional Info: You can use to compare characters (not Strings..
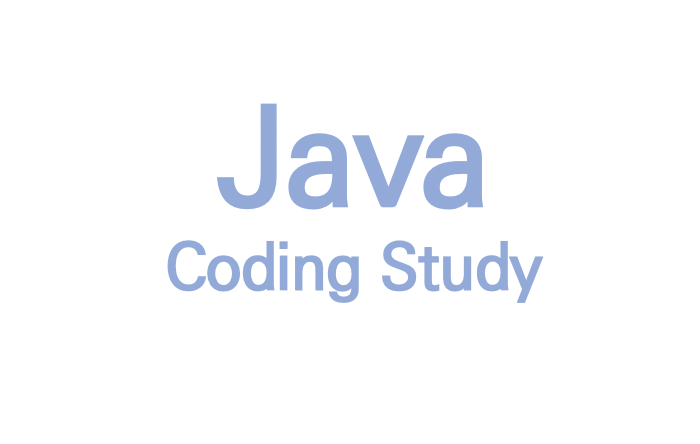
045 - Accumulator Method Practice 1
Write a method header on line two with the following specs: Returns: an integer Name: sumToX Parameters: an integer called "x" Purpose: calculate the sum of the integers from 1 to x (including x) Examples: sumToX(5) ==> 15 sumToX(7) ==> 28 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 import java.util.*; class Main { public static int sumToX(int x) { int sum=0; for(int i=1;i
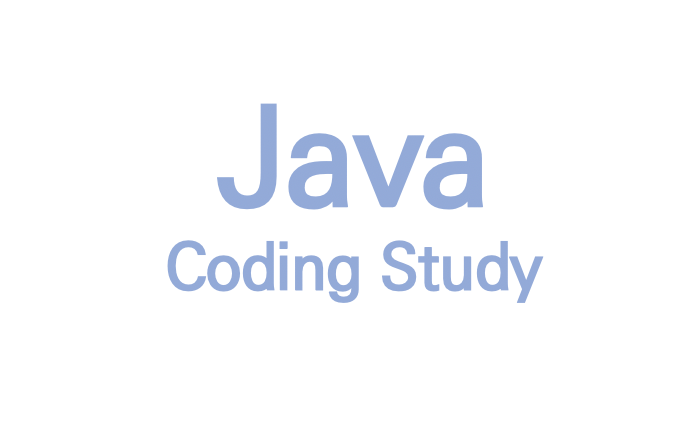
040 - Method Header Practice 5
Write a method header on line two with the following specs: Returns: a double Name: negate Parameters: a double called "num" Then, starting on line 4, write the code that will return the opposite value of num (if it's positive, make it negative, otherwise make it positive) Examples: 4 ==> -4 -10 ==> 10 1 ==> -1 Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 import java.util.*; class Main { public static..
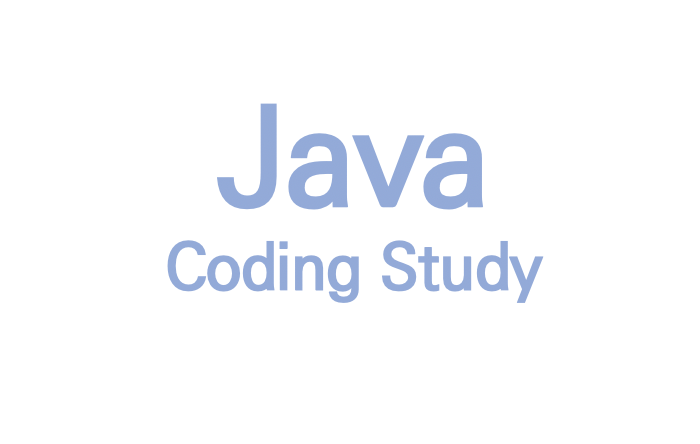
039 - Method Header Practice 4
Write a method header on line two with the following specs: Returns: an integer Name: addTwo Parameters: An integer called "x" An integer called "y" You should not be writing code on any line other than #2 1 2 3 4 5 6 7 8 9 10 11 12 import java.util.*; class Main { public static int addTwo(int x,int y){ return x+y; } //test case below (dont change): public static void main(String[] args){ System..
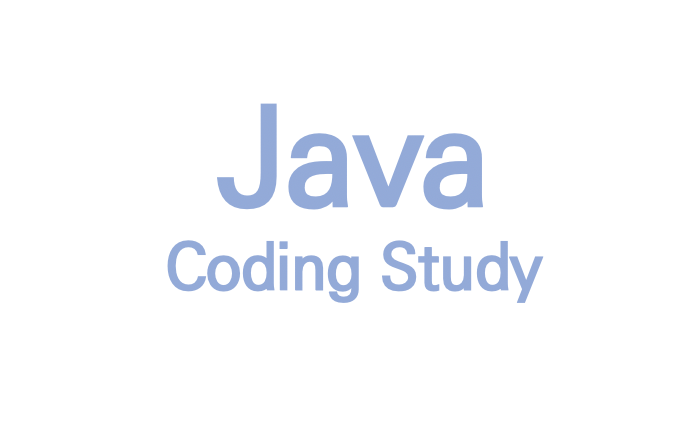
033 - For Loop Challenge 2 (optional)
Write code that will take in a String input and check to see if it is a palindrome or not. A palindrome means that the characters are the same forwards and backwards, ignoring spaces. Examples of palindromes: racecar was it a car or a cat I saw never odd or even rats live on no evil star Your check should be case insensitive too. For example, "Bob" is a palindrome, despite the first B being capi..
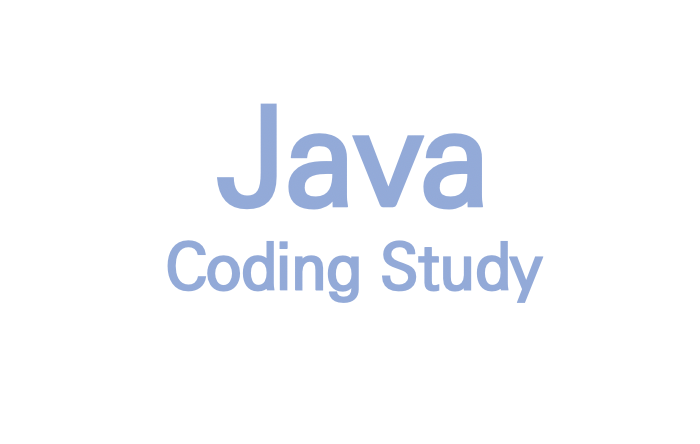
032 - For Loop Challenge 1 (optional)
The fibonacci sequence is a sequence of numbers in which the next number is the sum of the previous two numbers. The first two numbers of the fibonacci sequence are 0, 1. The first 8 numbers of the fibonacci sequence are 0, 1, 1, 2, 3, 5, 8, 13 Write some code to print out the first X numbers of the fibonacci sequence. Your output should be on one line, with each number separated by a space. You..
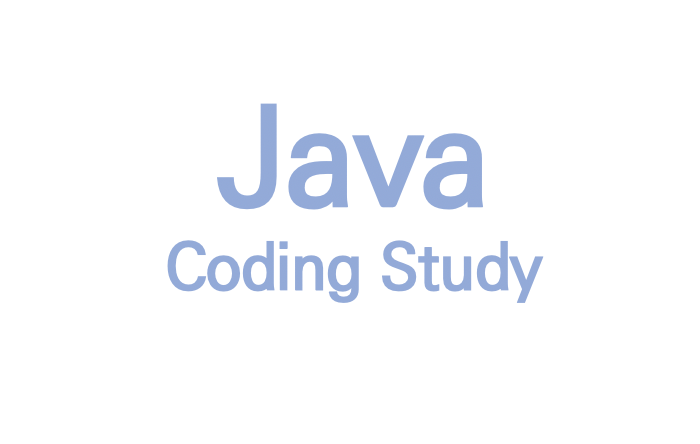
031 - Further For Loop Practice 7 (mIxEd CaSe)
Given the following inputs: String s; Write a for loop that will print out the string in alternating cases, with the first letter being lowercase. Keep in mind the following String methods: str.toUpperCase(); //make it uppercase str.toLowerCase(); //make it lowercase DO NOT USE .charAt()! .charAt returns a character, and you need a string in order to make it upper/lowercase. To get a letter at a..
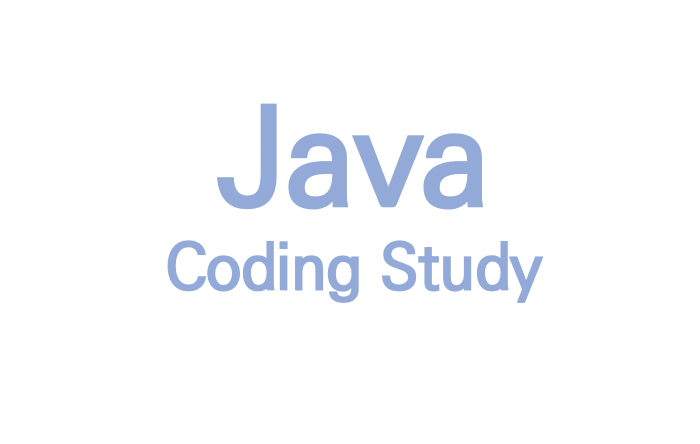
030 - Further For Loop Practice 6 (reverse string)
Given the following inputs: String s; Write a for loop that will print out the reverse of the string. Sample input/outputs: In: manhattan out: nattahnam In: processor out: rossecorp In: racecar out: racecar Solution 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; class Bakjoon { public static void main(String[] args) { Scanner inp = new Scanner(System.in); System.out.print("In:"); Str..